17.1 What are loops?
A loop is, very simply, code that tells a program like R to repeat a certain chunk of code several times with different values of a loop object that changes for every run of the loop. In R, the format of a for-loop is as follows:
# General structure of a loop
for(loop.object in loop.vector) {
LOOP.CODE
}
As you can see, there are three key aspects of loops: The loop object, the loop vector, and the loop code:
Loop object: The object that will change for each iteration of the loop. This is usually a letter like
i
, or an object with subscript likecolumn.i
orparticipant.i
. You can use any object name that you want for the index. While most people use single character object names, sometimes it’s more transparent to use names that tell you something about the data the object represents. For example, if you are doing a loop over participants in a study, you can call the indexparticipant.i
Loop vector: A vector specifying all values that the loop object will take over the loop. You can specify the values any way you’d like (as long as it’s a vector). If you’re running a loop over numbers, you’ll probably want to use
a:b
orseq()
. However, if you want to run a loop over a few specific values, you can just use thec()
function to type the values manually. For example, to run a loop over three different pirate ships, you could set the index values asship.i = c("Jolly Roger", "Black Pearl", "Queen Anne's Revenge")
.Loop code: The code that will be executed for all values in the loop vector. You can write any R code you’d like in the loop code - from plotting to analyses. R will run this code for all possible values of the loop object specified in the loop vector.
17.1.1 Printing numbers from 1 to 100
Let’s do a really simple loop that prints the integers from 1 to 10. For this code, our loop object is i
, our loop vector is 1:10
, and our loop code is print(i)
. You can verbally describe this loop as: For every integer i between 1 and 10, print the integer i:
# Print the integers from 1 to 10
for(i in 1:10) {
print(i)
}
## [1] 1
## [1] 2
## [1] 3
## [1] 4
## [1] 5
## [1] 6
## [1] 7
## [1] 8
## [1] 9
## [1] 10
As you can see, the loop applied the loop code (which in this case was print(i)
) to every value of the loop object i
specified in the loop vector
, 1:10
.
17.1.2 Adding the integers from 1 to 100
Let’s use a loop to add all the integers from 1 to 100. To do this, we’ll need to create an object called current.sum
that stores the latest sum of the numbers as we go through the loop. We’ll set the loop object to i
, the loop vector to 1:100
, and the loop code to current.sum <- current.sum + i
. Because we want the starting sum to be 0, we’ll set the initial value of current.sum
to 0. Here is the code:
# Loop to add integers from 1 to 100
current.sum <- 0 # The starting value of current.sum
for(i in 1:100) {
current.sum <- current.sum + i # Add i to current.sum
}
current.sum # Print the result!
## [1] 5050
Looks like we get an answer of 5050. To see if our loop gave us the correct answer, we can do the same calculation without a loop by using a:b
and the sum()
function:
# Add the integers from 1 to 100 without a loop
sum(1:100)
## [1] 5050
As you can see, the sum(1:100)
code gives us the same answer as the loop (and is much simpler).
There’s actually a funny story about how to quickly add integers (without a loop). According to the story, a lazy teacher who wanted to take a nap decided that the best way to occupy his students was to ask them to privately count all the integers from 1 to 100 at their desks. To his surprise, a young student approached him after a few moments with the correct answer: 5050. The teacher suspected a cheat, but the student didn’t count the numbers. Instead he realized that he could use the formula n(n+1) / 2
. Don’t believe the story? Check it out:
# Calculate the sum of integers from 1 to 100 using Gauss' method
n <- 100
n * (n + 1) / 2
## [1] 5050
This boy grew up to be Gauss, a super legit mathematician.
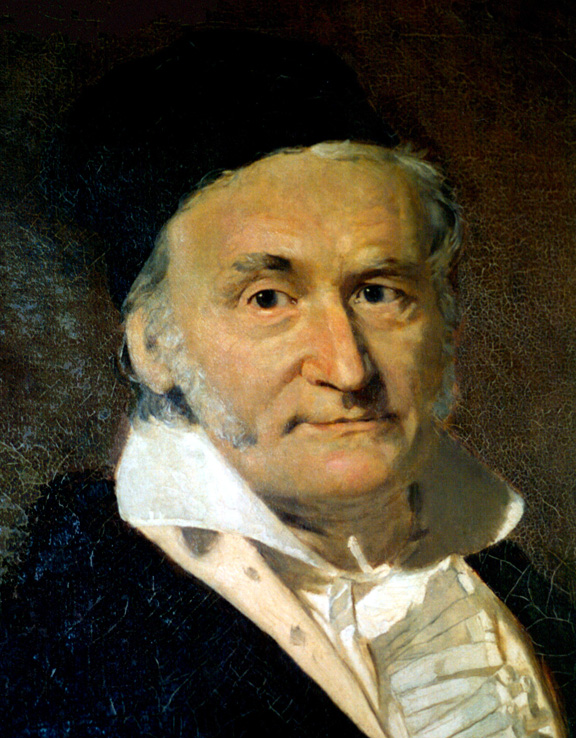
Figure 17.2: Gauss. The guy was a total pirate. And totally would give us shit for using a loop to calculate the sum of 1 to 100…