5.2 Vectors
Now let’s move onto vectors
. A vector object is just a combination of several scalars stored as a single object. For example, the numbers from one to ten could be a vector of length 10, and the characters in the English alphabet could be a vector of length 26. Like scalars, vectors can be either numeric or character (but not both!).
There are many ways to create vectors in R. Here are the methods we will cover in this chapter:
Function | Example | Result |
---|---|---|
c(a, b, ...) |
c(1, 5, 9) |
1, 5, 9 |
a:b |
1:5 |
1, 2, 3, 4, 5 |
seq(from, to, by, length.out) |
seq(from = 0, to = 6, by = 2) |
0, 2, 4, 6 |
rep(x, times, each, length.out) |
rep(c(7, 8), times = 2, each = 2) |
7, 7, 8, 8, 7, 7, 8, 8 |
The simplest way to create a vector is with the c()
function. The c here stands for concatenate, which means “bring them together”. The c()
function takes several scalars as arguments, and returns a vector containing those objects. When using c(), place a comma in between the objects (scalars or vectors) you want to combine:
Let’s use the c()
function to create a vector called a
containing the integers from 1 to 5.
# Create an object a with the integers from 1 to 5
a <- c(1, 2, 3, 4, 5)
# Print the result
a
## [1] 1 2 3 4 5
As you can see, R has stored all 5 numbers in the object a
. Thanks R!
You can also create longer vectors by combining vectors you have already defined. Let’s create a vector of the numbers from 1 to 10 by first generating a vector a
from 1 to 5, and a vector b
from 6 to 10 then combine them into a single vector x
:
a <- c(1, 2, 3, 4, 5)
b <- c(6, 7, 8, 9, 10)
x <- c(a, b)
x
## [1] 1 2 3 4 5 6 7 8 9 10
You can also create character vectors by using the c()
function to combine character scalars into character vectors:
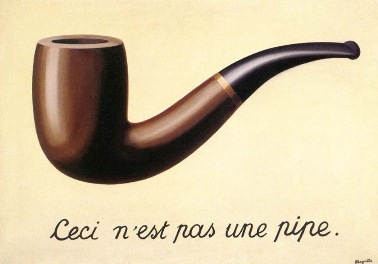
Figure 5.2: This is not a pipe. It is a character vector.
char.vec <- c("Ceci", "nest", "pas", "une", "pipe")
char.vec
## [1] "Ceci" "nest" "pas" "une" "pipe"
While the c()
function is the most straightforward way to create a vector, it’s also one of the most tedious. For example, let’s say you wanted to create a vector of all integers from 1 to 100. You definitely don’t want to have to type all the numbers into a c() operator. Thankfully, R has many simple built-in functions for generating numeric vectors. Let’s start with three of them: a:b
, seq()
, and rep()
:
5.2.1 a:b
The a:b
function takes two numeric scalars a
and b
as arguments, and returns a vector of numbers from the starting point a
to the ending point b
in steps of 1.
Here are some examples of the a:b
function in action. As you’ll see, you can go backwards or forwards, or make sequences between non-integers:
1:10
## [1] 1 2 3 4 5 6 7 8 9 10
10:1
## [1] 10 9 8 7 6 5 4 3 2 1
2.5:8.5
## [1] 2.5 3.5 4.5 5.5 6.5 7.5 8.5
5.2.2 seq()
Argument | Definition |
---|---|
from |
The start of the sequence |
to |
The end of the sequence |
by |
The step-size of the sequence |
length.out |
The desired length of the final sequence (only use if you don’t specify by ) |
The seq()
function is a more flexible version of a:b
. Like a:b
, seq()
allows you to create a sequence from a starting number to an ending number. However, seq()
, has additional arguments that allow you to specify either the size of the steps between numbers, or the total length of the sequence:
The seq()
function has two new arguments by
and length.out
. If you use the by
argument, the sequence will be in steps of the input to the by
argument:
# Create the numbers from 1 to 10 in steps of 1
seq(from = 1, to = 10, by = 1)
## [1] 1 2 3 4 5 6 7 8 9 10
# Integers from 0 to 100 in steps of 10
seq(from = 0, to = 100, by = 10)
## [1] 0 10 20 30 40 50 60 70 80 90 100
If you use the length.out
argument, the sequence will have length equal to length.out
.
# Create 10 numbers from 1 to 5
seq(from = 1, to = 5, length.out = 10)
## [1] 1.0 1.4 1.9 2.3 2.8 3.2 3.7 4.1 4.6 5.0
# 3 numbers from 0 to 100
seq(from = 0, to = 100, length.out = 3)
## [1] 0 50 100
5.2.3 rep()
Argument | Definition |
---|---|
x |
A scalar or vector of values to repeat |
times |
The number of times to repeat x |
each |
The number of times to repeat each value within x |
length.out |
The desired length of the final sequence |
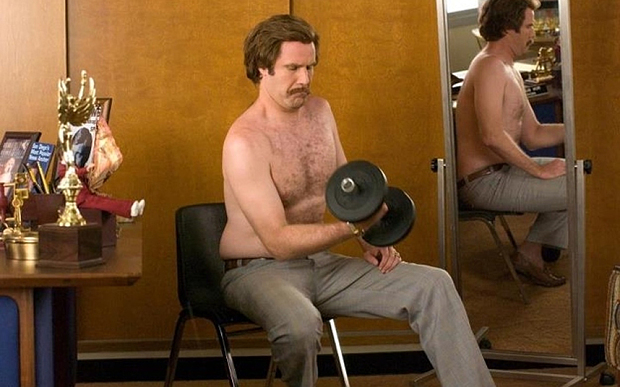
Figure 5.3: Not a good depiction of a rep in R.
The rep()
function allows you to repeat a scalar (or vector) a specified number of times, or to a desired length. Let’s do some reps.
rep(x = 3, times = 10)
## [1] 3 3 3 3 3 3 3 3 3 3
rep(x = c(1, 2), each = 3)
## [1] 1 1 1 2 2 2
rep(x = 1:3, length.out = 10)
## [1] 1 2 3 1 2 3 1 2 3 1
As you can see, you can can include an a:b
call within a rep()
!
You can even combine the times
and each
arguments within a single rep()
function. For example, here’s how to create the sequence {1, 1, 2, 2, 3, 3, 1, 1, 2, 2, 3, 3} with one call to rep()
:
rep(x = 1:3, each = 2, times = 2)
## [1] 1 1 2 2 3 3 1 1 2 2 3 3
Warning! Vectors contain either numbers or characters, not both
A vector can only contain one type of scalar: either numeric or character. If you try to create a vector with numeric and character scalars, then R will convert all of the numeric scalars to characters. In the next code chunk, I’ll create a new vector called my.vec
that contains a mixture of numeric and character scalars.
my.vec <- c("a", 1, "b", 2, "c", 3)
my.vec
## [1] "a" "1" "b" "2" "c" "3"
As you can see from the output, my.vec
is stored as a character vector where all the numbers are converted to characters.