2.3 Packages
When you download and install R for the first time, you are installing the Base R software. Base R will contain most of the functions you’ll use on a daily basis like mean()
and hist()
. However, only functions written by the original authors of the R language will appear here. If you want to access data and code written by other people, you’ll need to install it as a package. An R package is simply a bunch of data, from functions, to help menus, to vignettes (examples), stored in one neat package.
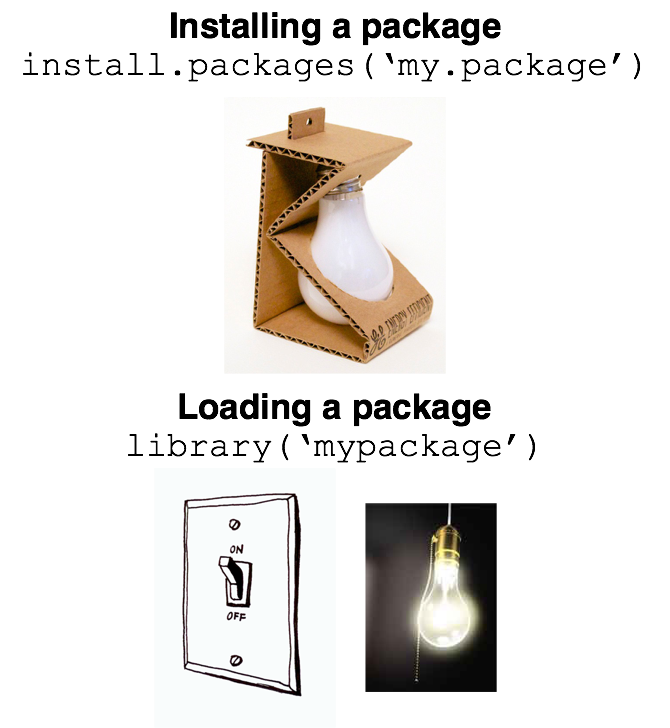
Figure 1.12: An R package is like a lightbulb. First you need to order it with install.packages(). Then, every time you want to use it, you need to turn it on with library()
A package is like a light bulb. In order to use it, you first need to order it to your house (i.e.; your computer) by installing it. Once you’ve installed a package, you never need to install it again. However, every time you want to actually use the package, you need to turn it on by loading it. Here’s how to do it.
2.3.1 Installing a new package
Installing a package simply means downloading the package code onto your personal computer. There are two main ways to install new packages. The first, and most common, method is to download them from the Comprehensive R Archive Network (CRAN). CRAN is the central repository for R packages. To install a new R package from CRAN, you can simply run the code install.packages("name")
, where “name” is the name of the package. For example, to download the yarrr
package, which contains several data sets and functions we will use in this book, you should run the following:
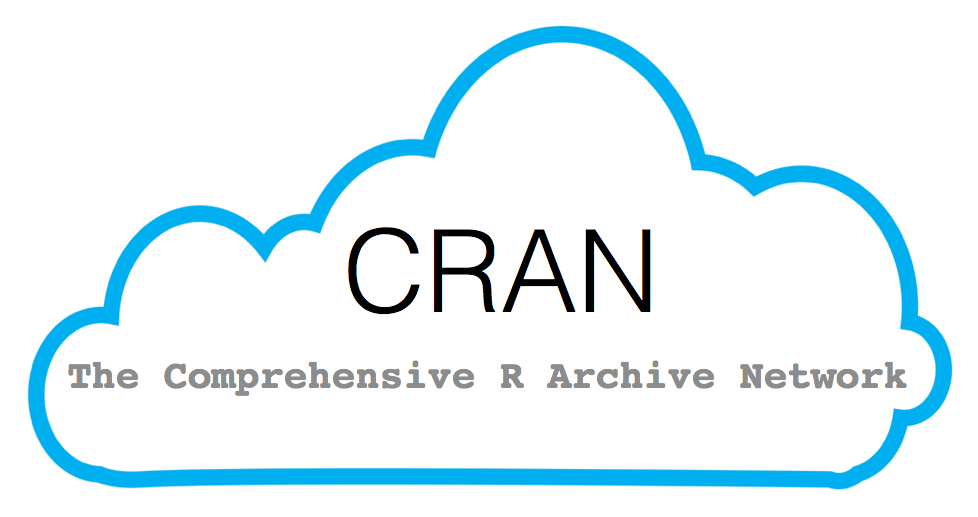
Figure 1.13: CRAN (Comprehensive R Archive Network) is the main source of R packages
# Install the yarrr package from CRAN
# You only need to install a package once!
install.packages("yarrr")
When you run install.packages("name")
R will download the package from CRAN. If everything works, you should see some information about where the package is being downloaded from, in addition to a progress bar.
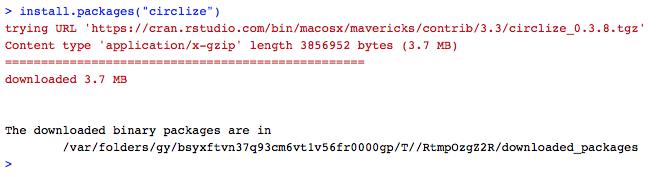
Figure 1.14: When you install a new package, you’ll see some random text like this you the download progress. You don’t need to memorize this.
Like ordering a light bulb, once you’ve installed a package on your computer you never need to install it again (unless you want to try to install a new version of the package). However, every time you want to use it, you need to turn it on by loading it.
2.3.2 Loading a package
Once you’ve installed a package, it’s on your computer. However, just because it’s on your computer doesn’t mean R is ready to use it. If you want to use something, like a function or dataset, from a package you always need to load the package in your R session first. Just like a light bulb, you need to turn it on to use it!
To load a package, you use the library()
function. For example, now that we’ve installed the yarrr
package, we can load it with library("yarrr")
:
# Load the yarrr package so I can use it!
# You have to load a package in every new R session!
library("yarrr")
Now that you’ve loaded the yarrr
package, you can use any of its functions! One of the coolest functions in this package is called pirateplot()
. Rather than telling you what a pirateplot is, let’s just make one. Run the following code chunk to make your own pirateplot. Don’t worry about the specifics of the code below, you’ll learn more about how all this works later. For now, just run the code and marvel at your pirateplot.
# Make a pirateplot using the pirateplot() function
# from the yarrr package!
pirateplot(formula = weight ~ Time,
data = ChickWeight,
pal = "xmen")
There is one way in R to temporarily load a package without using the library()
function. To do this, you can simply use the notation package::function
notation. This notation simply tells R to load the package just for this one chunk of code. For example, I could use the pirateplot
function from yarrr
package as follows:
# Use the pirateplot() function without loading the yarrr package first
yarrr::pirateplot(formula = weight ~ Diet,
data = ChickWeight)
Again, you can think about the package::function
method as a way to temporarily loading a package for a single line of code. One benefit of using the package::function
notation is that it’s immediately clear to anyone reading the code which package contains the function. However, a drawback is that if you are using a function from a package often, it forces you to constantly retype the package name. You can use whichever method makes sense for you.