17.3 Updating a container object with a loop
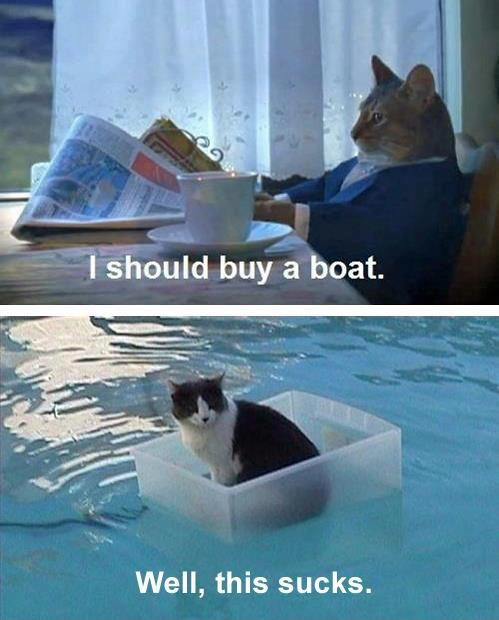
Figure 17.3: This is what I got when I googled ``funny container’’.
For many loops, you may want to update values of a ‘container’ object with each iteration of a loop. We can easily do this using indexing and assignment within a loop.
Let’s do an example with the examscores
dataframe. We’ll use a loop to calculate how many students failed each of the 4 exams – where failing is a score less than 50. To do this, we will start by creating an NA vector called failure.percent
. This will be a container object that we’ll update later with the loop.
# Create a container object of 4 NA values
failure.percent <- rep(NA, 4)
We will then use a loop that fills this object with the percentage of failures for each exam. The loop will go over each column in examscores
, calculates the percentage of scores less than 50 for that column, and assigns the result to the ith value of failure.percent
. For the loop, our loop object will be i
and our loop vector will be 1:4
.
for(i in 1:4) { # Loop over columns 1 through 4
# Get the scores for the ith column
x <- examscores[,i]
# Calculate the percent of failures
failures.i <- mean(x < 50)
# Assign result to the ith value of failure.percent
failure.percent[i] <- failures.i
}
Now let’s look at the result.
failure.percent
## [1] 0.50 1.00 0.03 0.97
It looks like about 50% of the students failed exam 1, everyone (100%) failed exam 2, 3% failed exam 3, and 97% percent failed exam 4. To calculate failure.percent
without a loop, we’d do the following:
# Calculate failure percent without a loop
failure.percent <- rep(NA, 4)
failure.percent[1] <- mean(examscores[,1] < 50)
failure.percent[2] <- mean(examscores[,2] < 50)
failure.percent[3] <- mean(examscores[,3] < 50)
failure.percent[4] <- mean(examscores[,4] < 50)
failure.percent
## [1] 0.50 1.00 0.03 0.97
As you can see, the results are identical.