2.5 Debugging
When you are programming, you will always, and I do mean always, make errors (also called bugs) in your code. You might misspell a function, include an extra comma, or some days…R just won’t want to work with you (again, see section Why R is like a Relationship).
Debugging will always be a challenge. However, over time you’ll learn which bugs are the most common and get faster and faster at finding and correcting them.
Here are the most common bugs you’ll run into as you start your R journey.
2.5.1 R is not ready (>)
Another very common problem occurs when R does not seem to be responding to your code. That is, you might run some code like mean(x)
expecting some output, but instead, nothing happens. This can be very frustrating because, rather than getting an error, just nothing happens at all. The most common reason for this is because R isn’t ready for new code, instead, it is waiting for you to finish code you started earlier, but never properly finished.
Think about it this way, R can be in one of two states: it is either Ready (>) for new code, or it is Waiting (+) for you to finish old code. To see which state R is in, all you have to do is look at the symbol on the console. The >
symbol means that R is Ready for new code – this is usually what you want to see. The +
symbol means that R is Waiting for you to (properly) finish code you started before. If you see the +
symbol, then no matter how much new code you write, R won’t actually evaluate it until you finish the code you started before.
Thankfully there is an easy solution to this problem (See Figure 1.15): Just hit the escape key on your keyboard. This will cancel R’s waiting state and make it Ready!
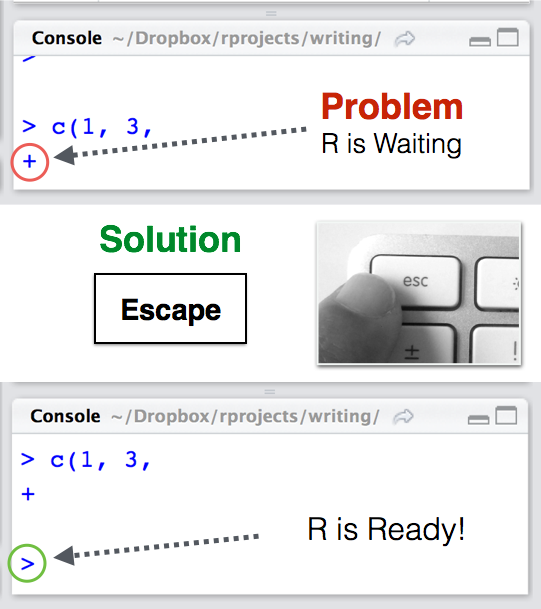
Figure 1.15: To turn R from a Waiting (+) state to a Ready (>) state, just hit Escape.
2.5.2 Misspelled object or function
If you spell an object or function incorrectly, you’ll receive an error like Error: could not find function
or Error: object 'x' not found
.
In the code below, I’ll try to take the mean of a vector data
, but I will misspell the function mean()
data <- c(1, 4, 3, 2, 1)
# Misspelled function: should be mean(x), not meeen(x)
meeen(data)
Now, I’ll misspell the object data
as dta
:
# Misspelled object: should be data, not dta
mean(dta)
R is case-sensitive, so if you don’t use the correct capitalization you’ll receive an error. In the code below, I’ll use Mean()
instead of the correct version mean()
# Capitalization is wrong: should be mean(), not Mean()
Mean(data)
Here is the correct version where both the object data
and function mean()
are correctly spelled:
# Correct: both the object and function are correctly spelled
mean(data)
## [1] 2.2
2.5.3 Punctuation problems
Another common error is having bad coding “punctuation”. By that, I mean having an extra space, missing a comma, or using a comma (,) instead of a period (.). In the code below, I’ll try to create a vector using periods instead of commas:
# Wrong: Using periods (.) instead of commas (,)
mean(c(1. 4. 2))
Because I used periods instead of commas, I get the above error. Here is the correct version
# Correct
mean(c(1, 4, 2))
## [1] 2.3
If you include an extra space in the middle of the name of an object or function, you’ll receive an error. In the code below, I’ll accidentally write Chick Weight
instead of ChickWeight
:
# Wrong: Extra space in the ChickWeight object name
head(Chick Weight)
Because I had an extra space in the object name, I get the above error. Here is the correction:
# Correct:
head(ChickWeight)