Chapter 2 Integration
Integration is the term we use to describe adding together an infinite number of infinitesimally small bits of something to make up the whole. For instance, in order to work out the length of a curve, we might break it into a lot of straight lines, and then add the lengths of these lines together:
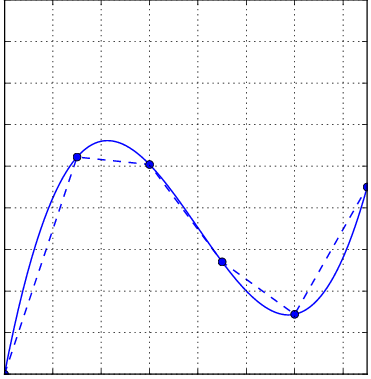
In the next bit of code we estimate the length of a semi circle of radius 1. What answer should we expect?
In the next bit of code we estimate the length of a semi circle of radius 1. What answer should we expect?
semicirc <-function(t){
(1-t^2)^(1/2)
}
npts <- 8 # as.integer(readline(prompt="Number of points:"))
div<-2/npts
x=seq(-1,1, by=div)
y=x[0:npts+1]
plot(y,semicirc(y),main="f(x)=sqr(1-x^2)",
ylab="f(x)",
type="l",
col="blue")
lentot<-0
for (i in 1:npts){
lentot<-lentot+((y[i+1]-y[i])^2+(semicirc(y[i+1])-semicirc(y[i]))^2)^(1/2)
}
print(lentot)
## [1] 3.104496
2.1 Integration of Polynomials
In this section we will look at the integration of polynomials by approximating the area under the curve by rectangles. Now let us think about the function on the interval , for some .
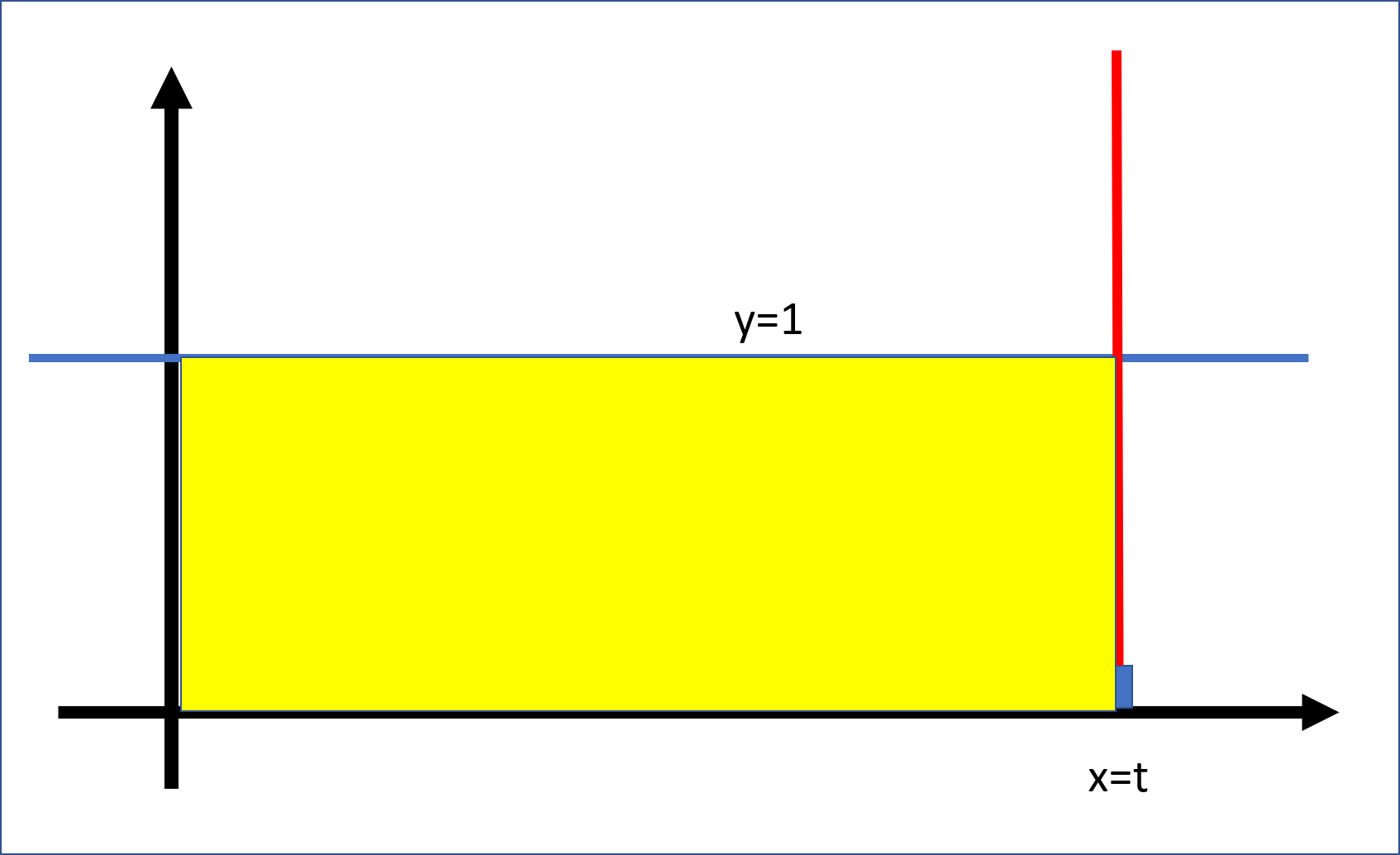
Then the area of the rectangle is and we say the integral of between and is , and we write
Now let us consider the function on the interval , for some . Here is a picture.
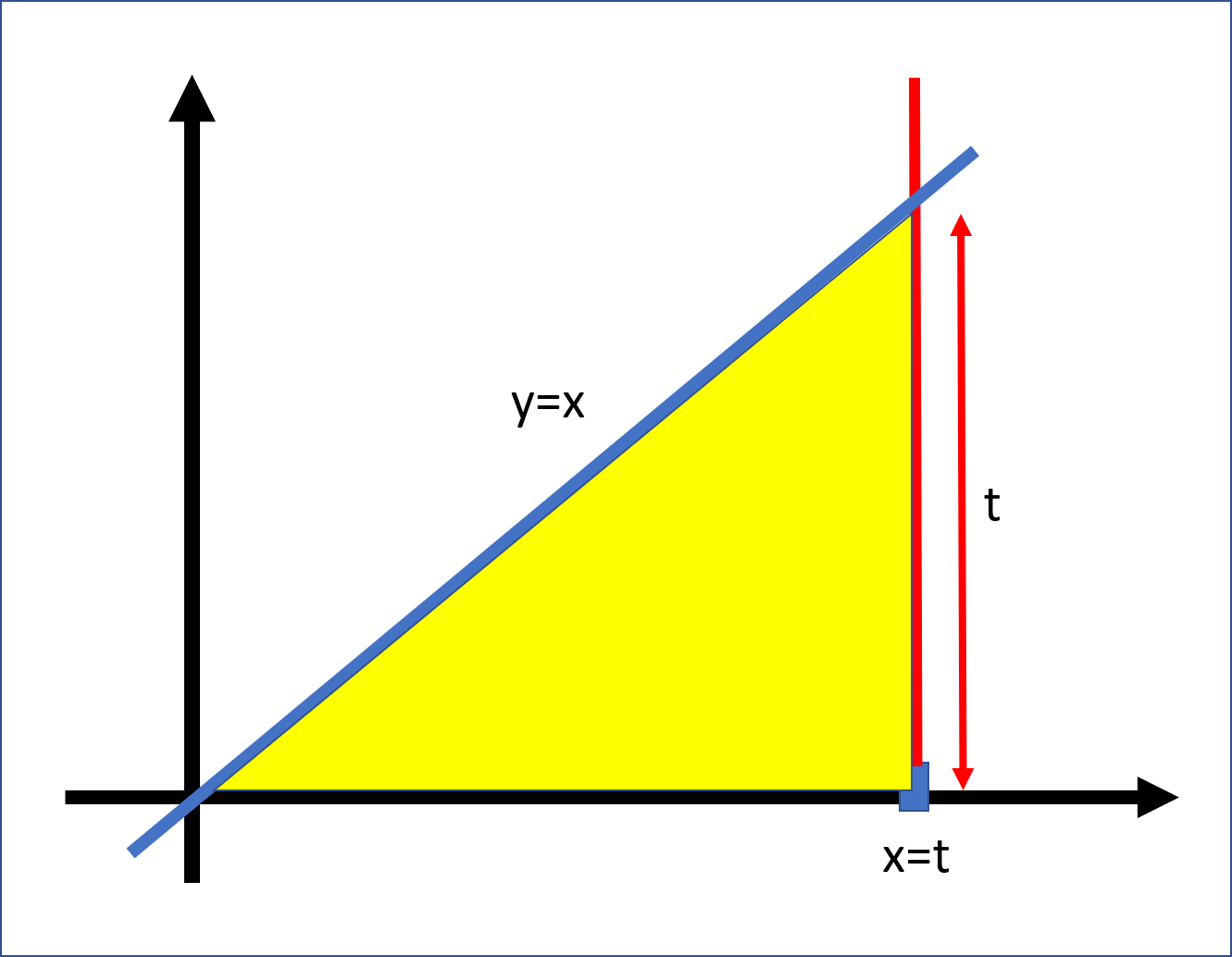
It is clear that the area of this is the area of an isosceles right-angled triangle of side length which is . Hence, the integral of between and is . We write this
Unfortunately, integrating other functions is not so straightforward. The next bit of code shows how to compute an esimate to the area under using rectangles.
parab <-function(x){
x^2
}
npts <- 16 # as.integer(readline(prompt="Number of points:"))
div<-1/npts
x <- seq(0,1,0.01)
plot(x,parab(x),main="f(x)=x^2",
ylab="f(x)",
type="l",
col="blue")
area<-0
for (i in 1:npts){
area<-area+(i-1)^2/npts^3
rect((i-1)/npts,0,i/npts,(i-1)^2/npts^2,col="red")
}
## [1] 0.3027344
Proof: Let us divide the interval into equal parts, each of width . Let us write , . Then the height of the th rectangle is (the left hand end of the first rectangle is ). If we substitute , we get the height . Hence the area of the th rectangle is Thus the total area of the rectangles is
For the moment we will just use the known fact that to see that
Substituting the last equation intp we get Now, as the second and third terms tend to zero (we will make these terms more precise later in the course), leaving us with the area
2.1.1 The cultural heritage of mathematics
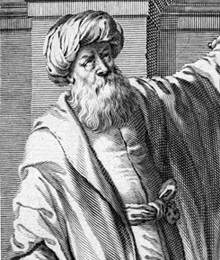
Hasan Ibn al-Haytham discovered the sum formula for the fourth power, using a method that could be generally used to determine the sum for any integral power. He used this to find the volume of a paraboloid. He could find the integral formula for any polynomial without having developed a general formula.
2.1.2 Test Yourself
Now you can test your understanding of integration with the following question. Put your answers in to 2 decimal places.
2.2 Challenge yourself
- Show that
- Use Q2 from Section 1.5 to show that for any
- Let be the rectangle rule estimate to using the left hand end rectangles. Let be the estimate using the right hand rectangles. Show that there is a positive constant such that Explain why is a better estimate than either or .
- Let be the estimate above using the height of the rectange at the middle of the interval as opposed to the left or right end. Show that there is a positive constant Explain why is a better estimate than or . Is it a better esimtate than ?