Dates and Times with lubridate
library(tidyverse)
library(lubridate)
library(nycflights13)
#today()
#now()
#from strings
ymd("2017-01-31")
## [1] "2017-01-31"
## [1] "2017-01-31"
mdy("January 31st, 2017")
## [1] "2017-01-31"
#mdy("Jan third, 2017")
mdy("Jan 03, 2017")
## [1] "2017-01-03"
## [1] "2017-01-03"
#mdy("Jan one, 2017")
dmy("31 Jan 2017")
## [1] "2017-01-31"
## [1] "2017-01-30 20:11:59 UTC"
mdy_hm("10/31/2017 08:01")
## [1] "2017-10-31 08:01:00 UTC"
## [1] "2017-01-31 UTC"
#from individual components
flights%>%
select(year, month, day, hour, minute)
## # A tibble: 336,776 x 5
## year month day hour minute
## <int> <int> <int> <dbl> <dbl>
## 1 2013 1 1 5 15
## 2 2013 1 1 5 29
## 3 2013 1 1 5 40
## 4 2013 1 1 5 45
## 5 2013 1 1 6 0
## 6 2013 1 1 5 58
## 7 2013 1 1 6 0
## 8 2013 1 1 6 0
## 9 2013 1 1 6 0
## 10 2013 1 1 6 0
## # … with 336,766 more rows
flights%>%
select(year, month, day, hour, minute)%>%
mutate(departure=make_datetime(year, month, day, hour, minute))
## # A tibble: 336,776 x 6
## year month day hour minute departure
## <int> <int> <int> <dbl> <dbl> <dttm>
## 1 2013 1 1 5 15 2013-01-01 05:15:00
## 2 2013 1 1 5 29 2013-01-01 05:29:00
## 3 2013 1 1 5 40 2013-01-01 05:40:00
## 4 2013 1 1 5 45 2013-01-01 05:45:00
## 5 2013 1 1 6 0 2013-01-01 06:00:00
## 6 2013 1 1 5 58 2013-01-01 05:58:00
## 7 2013 1 1 6 0 2013-01-01 06:00:00
## 8 2013 1 1 6 0 2013-01-01 06:00:00
## 9 2013 1 1 6 0 2013-01-01 06:00:00
## 10 2013 1 1 6 0 2013-01-01 06:00:00
## # … with 336,766 more rows
make_datetime_100 <- function(year, month, day, time){
make_datetime(year, month, day, time%/%100, time%%100)
}
(flights_dt <- flights %>%
filter(!is.na(dep_time), !is.na(arr_time))%>%
mutate(dep_time=make_datetime_100(year, month, day, dep_time),
arr_time=make_datetime_100(year, month, day, arr_time),
sched_dep_time=make_datetime_100(year, month, day, sched_arr_time))%>%
select(origin, dest, ends_with("delay"), ends_with("time")))
## # A tibble: 328,063 x 9
## origin dest dep_delay arr_delay dep_time sched_dep_time arr_time sched_arr_time air_time
## <chr> <chr> <dbl> <dbl> <dttm> <dttm> <dttm> <int> <dbl>
## 1 EWR IAH 2 11 2013-01-01 05:17:00 2013-01-01 08:19:00 2013-01-01 08:30:00 819 227
## 2 LGA IAH 4 20 2013-01-01 05:33:00 2013-01-01 08:30:00 2013-01-01 08:50:00 830 227
## 3 JFK MIA 2 33 2013-01-01 05:42:00 2013-01-01 08:50:00 2013-01-01 09:23:00 850 160
## 4 JFK BQN -1 -18 2013-01-01 05:44:00 2013-01-01 10:22:00 2013-01-01 10:04:00 1022 183
## 5 LGA ATL -6 -25 2013-01-01 05:54:00 2013-01-01 08:37:00 2013-01-01 08:12:00 837 116
## 6 EWR ORD -4 12 2013-01-01 05:54:00 2013-01-01 07:28:00 2013-01-01 07:40:00 728 150
## 7 EWR FLL -5 19 2013-01-01 05:55:00 2013-01-01 08:54:00 2013-01-01 09:13:00 854 158
## 8 LGA IAD -3 -14 2013-01-01 05:57:00 2013-01-01 07:23:00 2013-01-01 07:09:00 723 53
## 9 JFK MCO -3 -8 2013-01-01 05:57:00 2013-01-01 08:46:00 2013-01-01 08:38:00 846 140
## 10 LGA ORD -2 8 2013-01-01 05:58:00 2013-01-01 07:45:00 2013-01-01 07:53:00 745 138
## # … with 328,053 more rows
flights_dt%>%
ggplot(aes(dep_time))+
geom_freqpoly(binwidth=86400)
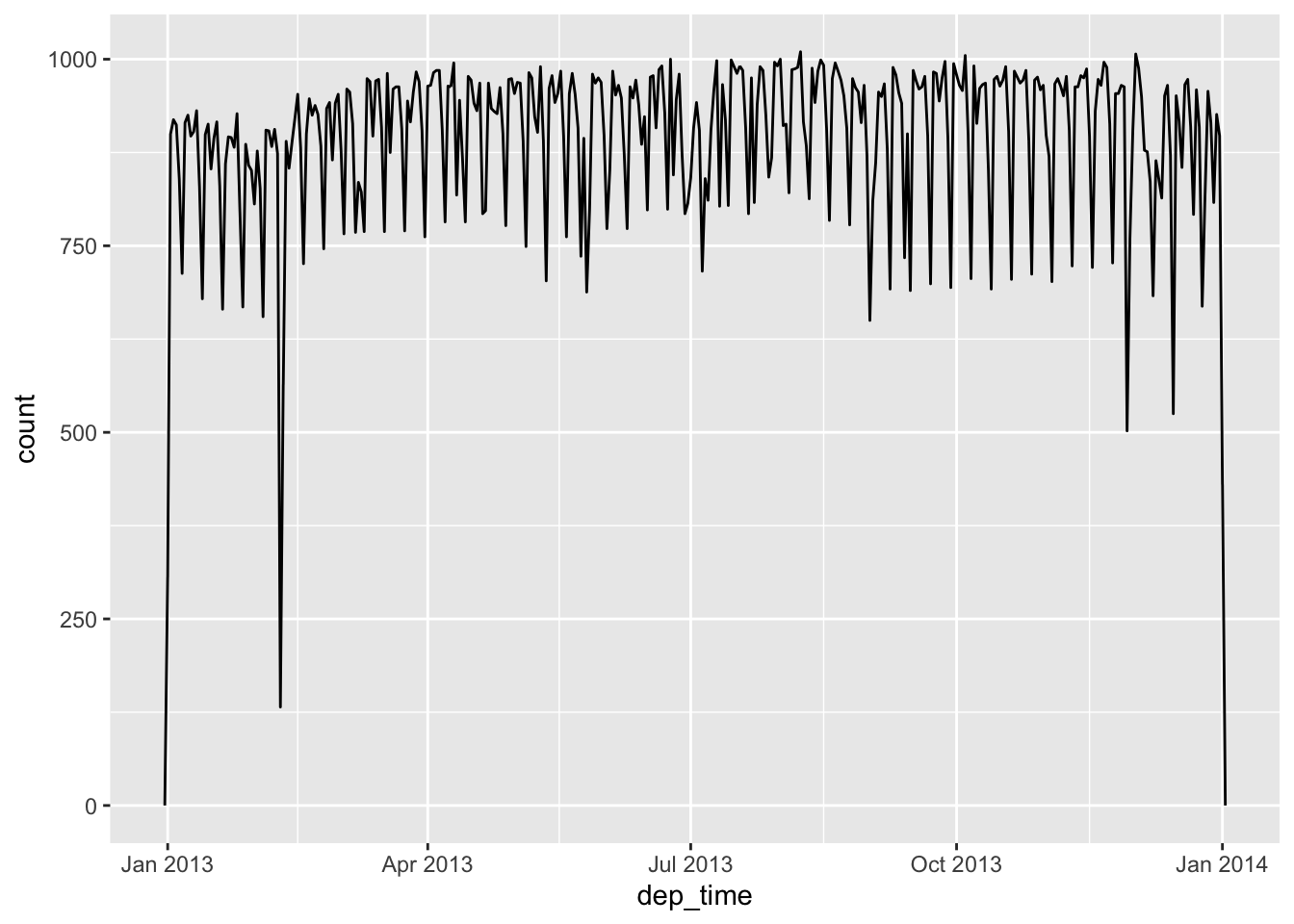
flights_dt%>%
filter(dep_time<ymd(20130102))%>%
ggplot(aes(dep_time))+
geom_freqpoly(binwidth=600)
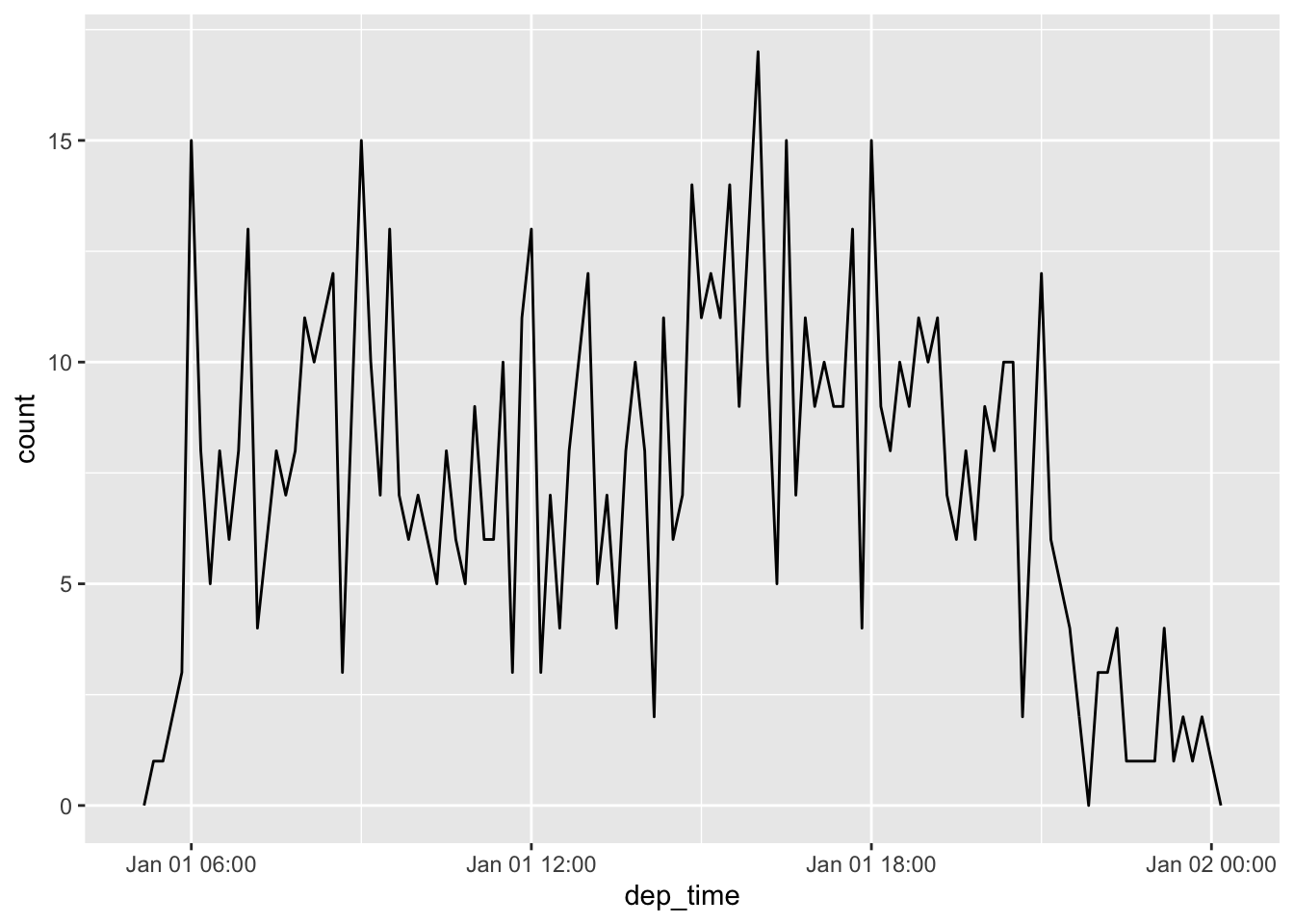