9.5 .RData files
The best way to store objects from R is with .RData files
. .RData
files are specific to R and can store as many objects as you’d like within a single file. Think about that. If you are conducting an analysis with 10 different dataframes and 5 hypothesis tests, you can save all of those objects in a single file called ExperimentResults.RData
.
9.5.1 save()
To save selected objects into one .RData
file, use the save()
function. When you run the save()
function with specific objects as arguments, (like save(a, b, c, file = "myobjects.RData"
) all of those objects will be saved in a single file called myobjects.RData
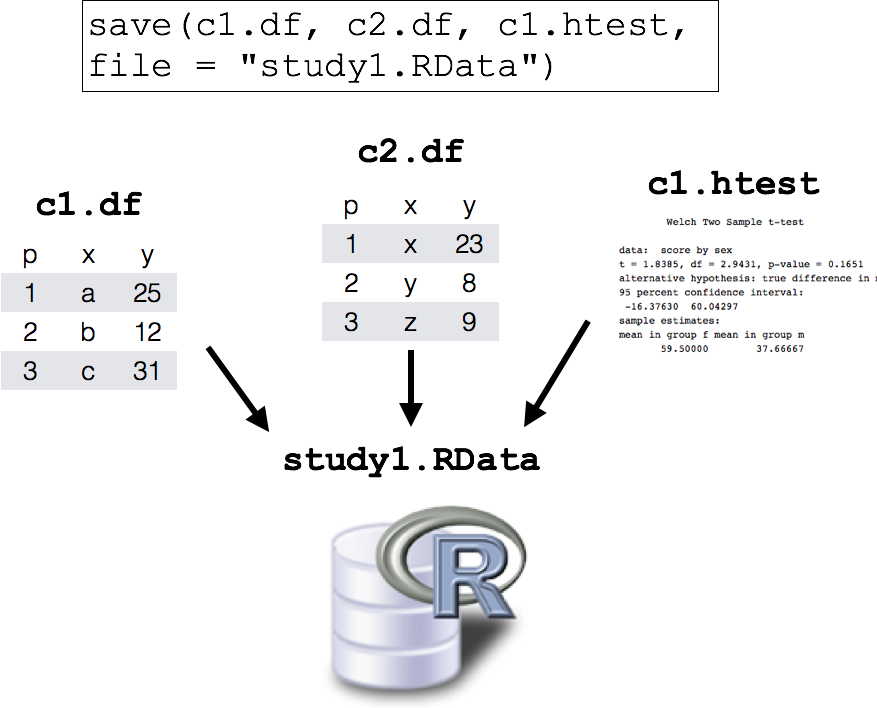
Figure 9.5: Saving multiple objects into a single .RData file.
For example, let’s create a few objects corresponding to a study.
# Create some objects that we'll save later
study1.df <- data.frame(id = 1:5,
sex = c("m", "m", "f", "f", "m"),
score = c(51, 20, 67, 52, 42))
score.by.sex <- aggregate(score ~ sex,
FUN = mean,
data = study1.df)
study1.htest <- t.test(score ~ sex,
data = study1.df)
Now that we’ve done all of this work, we want to save all three objects in an a file called study1.RData
in the data folder of my current working directory. To do this, you can run the following
# Save two objects as a new .RData file
# in the data folder of my current working directory
save(study1.df, score.by.sex, study1.htest,
file = "data/study1.RData")
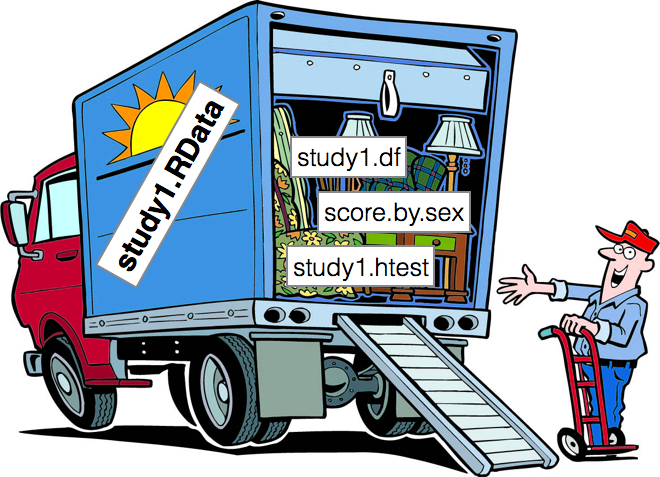
Figure 9.6: Our new study1.RData file is like a van filled with our objects.
Once you do this, you should see the study1.RData
file in the data folder of your working directory. This file now contains all of your objects that you can easily access later using the load()
function (we’ll go over this in a second…).
9.5.2 save.image()
If you have many objects that you want to save, then using save
can be tedious as you’d have to type the name of every object. To save all the objects in your workspace as a .RData file, use the save.image()
function. For example, to save my workspace in the data
folder located in my working directory, I’d run the following:
# Save my workspace to complete_image.RData in th,e
# data folder of my working directory
save.image(file = "data/projectimage.RData")
Now, the projectimage.RData
file contains all objects in your current workspace.
9.5.3 load()
To load an .RData
file, that is, to import all of the objects contained in the .RData
file into your current workspace, use the load()
function. For example, to load the three specific objects that I saved earlier (study1.df
, score.by.sex
, and study1.htest
) in study1.RData
, I’d run the following:
# Load objects in study1.RData into my workspace
load(file = "data/study1.RData")
To load all of the objects in the workspace that I just saved to the data folder in my working directory in projectimage.RData
, I’d run the following:
# Load objects in projectimage.RData into my workspace
load(file = "data/projectimage.RData")
I hope you realize how awesome loading .RData files is. With R, you can store all of your objects, from dataframes to hypothesis tests, in a single .RData
file. And then load them into any R session at any time using load()
.
9.5.4 rm()
To remove objects from your workspace, use the rm()
function. Why would you want to remove objects? At some points in your analyses, you may find that your workspace is filled up with one or more objects that you don’t need – either because they’re slowing down your computer, or because they’re just distracting.
To remove specific objects, enter the objects as arguments to rm()
. For example, to remove a huge dataframe called huge.df
, I’d run the following;
# Remove huge.df from workspace
rm(huge.df)
If you want to remove all of the objects in your working directory, enter the argument list = ls()
# Remove ALL objects from workspace
rm(list = ls())
Important!!! Once you remove an object, you cannot get it back without running the code that originally generated the object! That is, you can’t simply click ‘Undo’ to get an object back. Thankfully, if your R code is complete and well-documented, you should easily be able to either re-create a lost object (e.g.; the results of a regression analysis), or re-load it from an external file.