4 Determining the best fitting models.
4.1 Energy Predictions.
The regression analysis conducted returns 3 coefficients for each model created, these can be accessed via the following code,
# List of Modelling Coefficients.
<- coeffMatrix[1, 1]
Base <- coeffMatrix[2, 1]
Slope <- coeffMatrix[3, 1] Intercept
An energy signature prediction can then be made using the following formula,
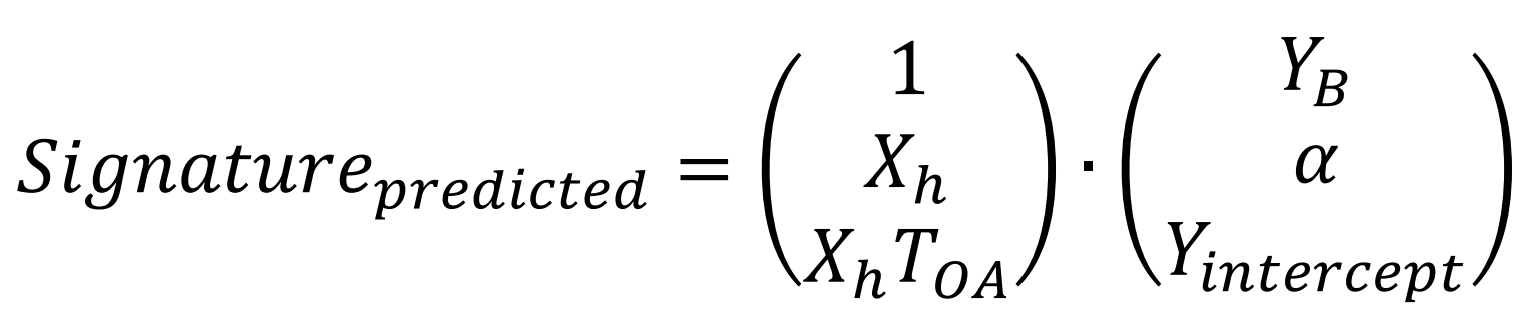
Figure 4.1: Formula for energy predictions
Where YB, \(\alpha\) and Yintercept are the Base, Slope and Intercept coefficients respectively.
This is represented in the code by the following,
# Energy Signature Prediction.
<- Base + (Slope * Dummy_Temp) + (Intercept * Dummy_Variables) Sig_Pred
Then by accessing the number of days in each month and multiplying by 24,
the hours per month is obtained.
This can be used with energy signature predictions to create total
consumption predictions.
# Hours in a given Month.
<- Current_Set$Days.in.the.Month * 24
Hours_Month
# Energy Prediction.
<- Sig_Pred * Hours_Month Energy_Pred
Finally, subtracting the predicted consumption away from the measured consumption gives a column of residuals, which will be used to generate the statistical indicators.
# Calculates the Energy Residuals.
<- Energy_Measured - Energy_Pred Residuals
4.2 Statistical Indicators.
Well-calibrated building energy models should meet guidelines for at least 4 different statistical indicators, namely Root Mean Square Error [RMSE], Coefficient of variation of RMSE [CV(RMSE)], Coefficient of determination [R2] and Normalised mean bias error [NMBE].
Variance can be calculated with a base R function, the statistical indicators are then calculated with the following formulae,
# Variance (Sample).
<- var(Energy_Measured)
Var
# Calculating RMSE, (the 3 represents the degrees of freedom).
<- (sum(Residuals^2) / (nTrain - 3))^0.5
RMSE
# Coefficient of Variation of Root Mean Square Error.
<- 100 * (nTrain * RMSE) / sum(Energy_Measured)
CV_RMSE
# Coefficient of Determination R^2.
<- 100 * (1 - ((sum(Residuals^2)) / (nTrain * Var)))
R2
# Normalised mean bias error (NMBE).
<- 100 * ((sum(Residuals) * nTrain) / ((nTrain - 3) * sum(Energy_Measured))) NMBE
4.3 Storing the best-fitting models.
Finally, to conclude this section the best fitting balance point temperatures need
to be isolated.
This is completed in three steps:
1. Add the current data to a comparison table and remove the dummy variables
before the next temperature loop begins.
2. After the temperature loop has been completed, identify the row of data with the
best statistical indicators (in this case RMSE was used).
3. Insert the best model into a new list.
# Saves data in Comparison_Table for each Balance_Point.
1:10] <- c(
Comparison_Table[j, $id[j], Current_Set$Building.Name[j], Balance_Point,
Current_Set
Base, Intercept, Slope, RMSE, CV_RMSE, R2, NMBE)
# Removes the Dummy columns before the next loop.
<- Current_Set[, -13:-14]
Current_Set
} # End of Temperature Looping
# Identify the minimum RMSE.
<- which.min(Comparison_Table$V7)
n
# Selecting the best fitting values from the Comparison Table and store them in a Final List.
1:10] <- Comparison_Table[n, c("V1", "V2", "V3", "V4", "V5",
Best_Model_List[i, "V6", "V7", "V8", "V9", "V10")]