3 Creating the Energy Models.
3.1 Determining the number of loops.
Prior to beginning the first loop, “regData” can be analysed with the following code. This will eventually be used to tell the code in the following sections how many iterations to do.
# number of rows in the csv.
<- nrow(regData)
nData
# Arranging k separate sets for the 48 separate buildings.
<- 1:nData
rowNumbers <- max(na.omit(regData$K.SETS))
kSets
# Number of Balance points that need to be trialed.
<- length(BP_trial) nBP
3.2 Starting the Time Period Loop.
The chunk of code seen below is used to assign a split in the dataset so that energy models from each time period can be assessed separately.
Importantly, notice how there is an unmatched set of braces “{}”, this is because all of the code in sections 3.3 and 3.4 need to be contained and should be indented within this first loop.
for (x in 1:3) { # Loop 3 times, because there are 3 Time Periods in the example.
if (x == 1) {
<- Split_1
Current_Rows <- Title_1}
Period if (x == 2) {
<- Split_2
Current_Rows <- Title_2}
Period if (x == 3) {
<- Split_3
Current_Rows <- Title_3} Period
The “Current_Rows” will then be used in the following section to separate the
relevant data.
The strings saved in “Period”, are used for plotting at the end
of the building loop.
3.3 Seperating the Buildings for Looping.
A loop through all of the “K” sets can now be conducted, i.e., to create models
for each building separately.
First, we sort the relevant data so that only the current building “i” is modeled.
This can be achieved with the lines starting “trainSet” and “trainData”.
“Current_Set” further filters the data by the Time Period currently being looped.
“nTrain” is a variable that will be used later in the project.
Similarly, to the previous code chunk, the following code has another open brace,
this is because all of the next loop should be indented within this loop too.
# For each building 'i' in the dataset.
for (i in 1:kSets) {
# -------- Data selection --------
# Select the set rows which correspond to the current building 'i'.
<- subset(rowNumbers, regData$K.SETS == i)
trainSet
# Selects the data from the csv for modelling, according to the training rows.
<- regData[trainSet, ]
trainData
# Select the correct years of data only.
<- trainData[Current_Rows, ]
Current_Set
# Length of the Current set.
<- length(Current_Set) nTrain
3.4 Balance Point Testing.
At this stage of the code, we have isolated all of the relevant data and are ready to start creating the building energy models.
Explained as simply as possible, to create “3PH Heating models”, energy signatures will be regressed against a “dummy variable” and a list of the temperatures below the balance point.
The figure below showcases the logic to select dummy variables.
Where TOA is the outdoor air temperature, TBP is the
balance point temperature, and Xh is the dummy variable,
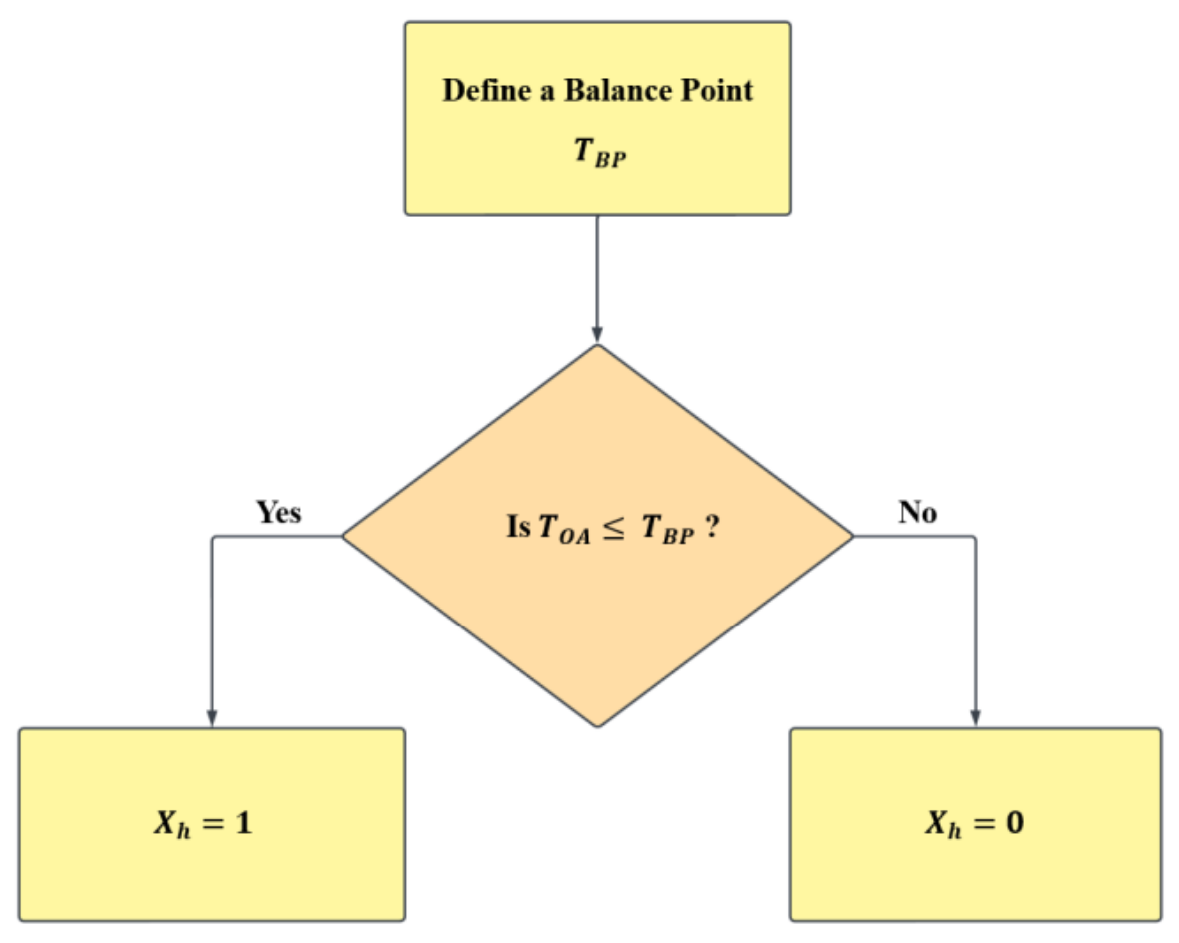
Figure 3.1: Dummy variable logic.
One final loop is created to test each potential “Balance Point”. For each, the
dummy variables are created using the recorded temperatures.
A list of the temperatures below the balance point can simply be created
by multiplying the Temperatures and the Dummy Variables.
Finally, these new columns are binded to the “Current_Set” of data.
# For each Balance Point 'j' that we are testing.
for (j in 1:nBP) {
# -------- Dummy Variables --------
# Selects the temperature column.
<- Current_Set$Temperature.C
Temperatures
# Assigns the current Balance Point that needs to be tested.
<- BP_trial[j]
Balance_Point
# Compares values against the Balance point.
# If a value is below the balance point, returns a 1,
# Else, returns a 0.
<- ifelse(Temperatures <= Balance_Point, 1, 0)
Dummy_Variables
# Creates the "modified temperature list", i.e., the temperature list with
# zeros in place of temperatures greater than the balance point.
<- Temperatures * Dummy_Variables
Dummy_Temp
# Adds the Dummy Variables and Dummy*Temp list from the current Balance Point
# to this the Training Data set.
<- cbind(Current_Set, Dummy_Variables, Dummy_Temp) Current_Set
3.5 Regression Analysis.
Finally, to end this section of the project, the regression models will be created.
These can simply be created using the linear model “lm” function.
The regression coefficients are then saved into the coefficient matrix.
# Creates the linear Regression models.
<- lm(Energy.Signature ~ Dummy_Temp + Dummy_Variables, data = Current_Set)
regModel
# Creating the Coefficient Matrix.
<- cbind(summary.lm(regModel)$coefficients) coeffMatrix