Day 2 Crawling the web
Today’s session will be dedicated to getting data from the web. This process is also called scraping since we scrape data off from the surface and remodel it for our inferences. The following picture shows you the web scraping cheat sheet that outlines the process of scraping the web. On the left side, you can see the first step in scraping the web which is requesting the information from the server. This is basically what is going under the hood when you make requests using a browser. The response is the website, usually stored in an XML document, which is then the starting point for your subsequent queries and data extraction.
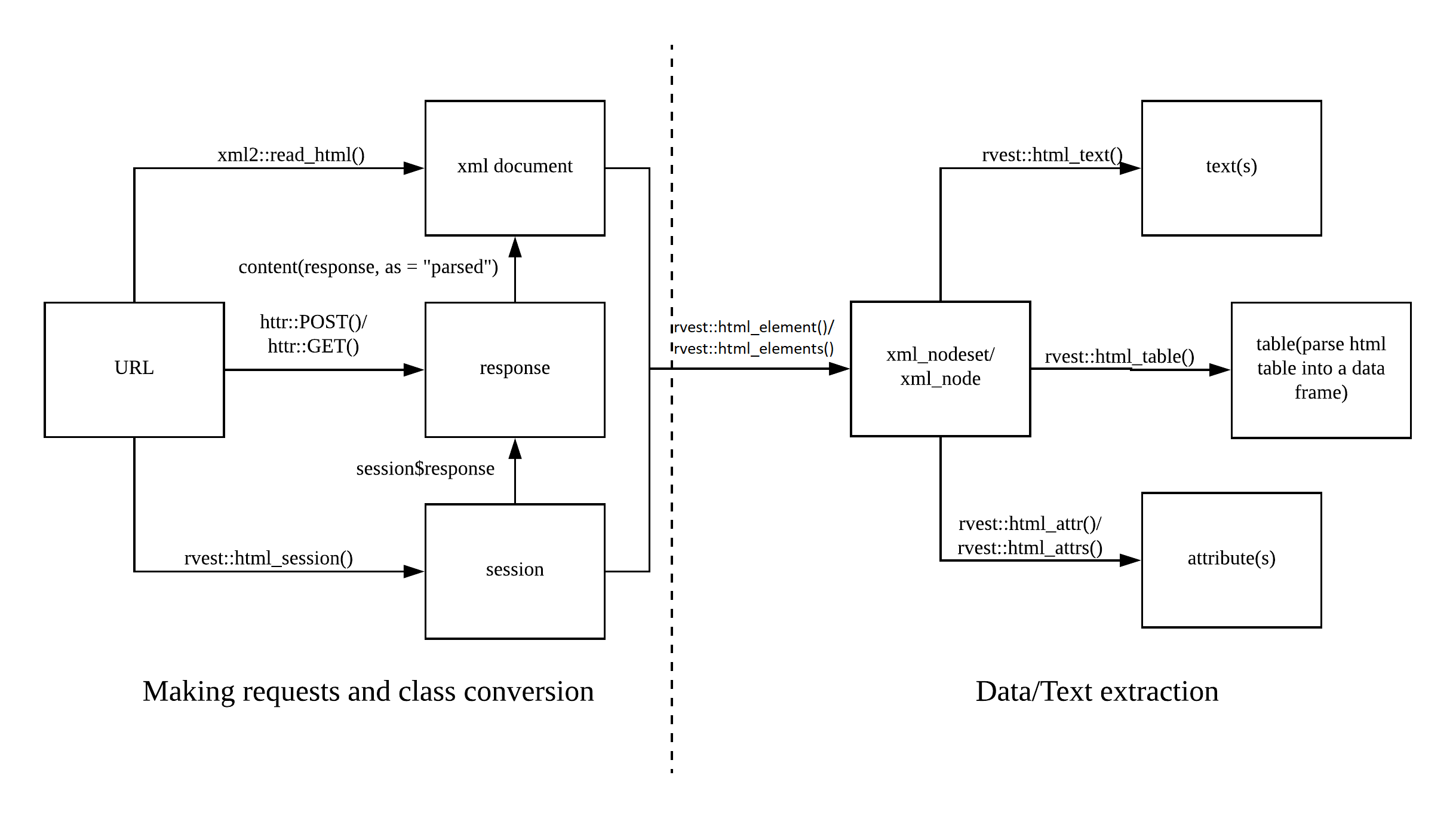
In today’s session, you will learn different techniques to get your hands on data. In particular, this will encompass making simple URL requests with read_html()
, using session()
s to navigate around on a web page, submitting html_form()
s to fill in forms on a web page, and making structured requests to APIs.
2.1 Getting started with rvest
2.1.1 Making requests
The most basic form of making a request is by using read_html()
from the xml2
package.
#install.packages("needs")
needs(httr, rvest, tidyverse)
page <- read_html("https://en.wikipedia.org/wiki/Tidyverse")
page %>% str()
## List of 2
## $ node:<externalptr>
## $ doc :<externalptr>
## - attr(*, "class")= chr [1:2] "xml_document" "xml_node"
This is perfectly fine for making requests to static pages where you do not need to take any further action. Sometimes, however, this is not enough, and you want to accept cookies or move on the page.
2.1.2 session()
s
However, the slickest way to do this is by using a session()
. In a session, R behaves like a normal browser, stores cookies, allows you to navigate between pages, by going session_forward()
or session_back()
, session_follow_link()
s on the page itself or session_jump_to()
a different URL, or submit form()
s with session_submit()
.
First, you start the session by simply calling session()
.
Some servers may not want robots to make requests and block you for this reason. To circumnavigate this, we can set a “user agent” in a session. The user agent contains data that the server receives from us when we make the request. Hence, by adapting it we can trick the server into thinking that we are humans instead of robots. Let’s check the current user agent first:
## [1] "libcurl/7.88.1 r-curl/5.0.1 httr/1.4.6"
Not very human. We can set it to a common one using the httr
package (which powers rvest
).
user_a <- user_agent("Mozilla/5.0 (Macintosh; Intel Mac OS X 12_0_1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.69 Safari/537.36")
session_with_ua <- session("https://scrapethissite.com/", user_a)
session_with_ua$response$request$options$useragent
## [1] "Mozilla/5.0 (Macintosh; Intel Mac OS X 12_0_1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/95.0.4638.69 Safari/537.36"
You can check the response using session$response$status_code
– 200 is good.
## [1] 200
When you want to save a page from the session, do so using read_html()
.
If you want to open a new URL, use session_jump_to()
.
session_with_ua <- session_with_ua |>
session_jump_to("https://www.scrapethissite.com/pages/")
session_with_ua
## <session> https://www.scrapethissite.com/pages/
## Status: 200
## Type: text/html; charset=utf-8
## Size: 10603
You can also click buttons on the page using CSS selectors or XPATHs (more on them tomorrow!):
session_with_ua <- session_with_ua |>
session_jump_to("https://www.scrapethissite.com/") |>
session_follow_link(css = ".btn-primary")
## Navigating to /lessons/
## <session> http://www.scrapethissite.com/lessons/sign-up/
## Status: 200
## Type: text/html; charset=utf-8
## Size: 24168
Wanna go back – session_back()
; thereafter you can go session_forward()
, too.
## <session> https://www.scrapethissite.com/
## Status: 200
## Type: text/html; charset=utf-8
## Size: 8117
## <session> http://www.scrapethissite.com/lessons/sign-up/
## Status: 200
## Type: text/html; charset=utf-8
## Size: 24168
You can look at what your scraper has done with session_history()
.
## https://www.scrapethissite.com/
## https://www.scrapethissite.com/pages/
## https://www.scrapethissite.com/
## - http://www.scrapethissite.com/lessons/sign-up/
2.1.3 Forms
Sometimes we also want to provide certain input, e.g., to provide login credentials or to scrape a website more systematically. That information is usually provided using so-called forms. A <form>
element can contain different other elements such as text fields or check boxes. Basically, we use html_form()
to extract the form, html_form_set()
to define what we want to submit, and html_form_submit()
to finally submit it. For a basic example, we search for something on Google.
google <- read_html("http://www.google.com")
search <- html_form(google) |> pluck(1)
search |> str()
## List of 5
## $ name : chr "f"
## $ method : chr "GET"
## $ action : chr "http://www.google.com/search"
## $ enctype: chr "form"
## $ fields :List of 10
## ..$ ie :List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "ie"
## .. ..$ value: chr "ISO-8859-1"
## .. ..$ attr :List of 3
## .. .. ..$ name : chr "ie"
## .. .. ..$ value: chr "ISO-8859-1"
## .. .. ..$ type : chr "hidden"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ hl :List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "hl"
## .. ..$ value: chr "fr"
## .. ..$ attr :List of 3
## .. .. ..$ value: chr "fr"
## .. .. ..$ name : chr "hl"
## .. .. ..$ type : chr "hidden"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ source:List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "source"
## .. ..$ value: chr "hp"
## .. ..$ attr :List of 3
## .. .. ..$ name : chr "source"
## .. .. ..$ type : chr "hidden"
## .. .. ..$ value: chr "hp"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ biw :List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "biw"
## .. ..$ value: NULL
## .. ..$ attr :List of 2
## .. .. ..$ name: chr "biw"
## .. .. ..$ type: chr "hidden"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ bih :List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "bih"
## .. ..$ value: NULL
## .. ..$ attr :List of 2
## .. .. ..$ name: chr "bih"
## .. .. ..$ type: chr "hidden"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ q :List of 4
## .. ..$ type : chr "text"
## .. ..$ name : chr "q"
## .. ..$ value: chr ""
## .. ..$ attr :List of 8
## .. .. ..$ class : chr "lst"
## .. .. ..$ style : chr "margin:0;padding:5px 8px 0 6px;vertical-align:top;color:#000"
## .. .. ..$ autocomplete: chr "off"
## .. .. ..$ value : chr ""
## .. .. ..$ title : chr "Recherche Google"
## .. .. ..$ maxlength : chr "2048"
## .. .. ..$ name : chr "q"
## .. .. ..$ size : chr "57"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ btnG :List of 4
## .. ..$ type : chr "submit"
## .. ..$ name : chr "btnG"
## .. ..$ value: chr "Recherche Google"
## .. ..$ attr :List of 4
## .. .. ..$ class: chr "lsb"
## .. .. ..$ value: chr "Recherche Google"
## .. .. ..$ name : chr "btnG"
## .. .. ..$ type : chr "submit"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ btnI :List of 4
## .. ..$ type : chr "submit"
## .. ..$ name : chr "btnI"
## .. ..$ value: chr "J'ai de la chance"
## .. ..$ attr :List of 5
## .. .. ..$ class: chr "lsb"
## .. .. ..$ id : chr "tsuid_1"
## .. .. ..$ value: chr "J'ai de la chance"
## .. .. ..$ name : chr "btnI"
## .. .. ..$ type : chr "submit"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ iflsig:List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "iflsig"
## .. ..$ value: chr "AOEireoAAAAAZJnGV4cPNCrV2ScdsDbpt_8NKI3gpPb3"
## .. ..$ attr :List of 3
## .. .. ..$ value: chr "AOEireoAAAAAZJnGV4cPNCrV2ScdsDbpt_8NKI3gpPb3"
## .. .. ..$ name : chr "iflsig"
## .. .. ..$ type : chr "hidden"
## .. ..- attr(*, "class")= chr "rvest_field"
## ..$ gbv :List of 4
## .. ..$ type : chr "hidden"
## .. ..$ name : chr "gbv"
## .. ..$ value: chr "1"
## .. ..$ attr :List of 4
## .. .. ..$ id : chr "gbv"
## .. .. ..$ name : chr "gbv"
## .. .. ..$ type : chr "hidden"
## .. .. ..$ value: chr "1"
## .. ..- attr(*, "class")= chr "rvest_field"
## - attr(*, "class")= chr "rvest_form"
search_something <- search |> html_form_set(q = "something")
resp <- html_form_submit(search_something, submit = "btnG")
read_html(resp)
## {html_document}
## <html lang="fr">
## [1] <head>\n<meta http-equiv="Content-Type" content="text/html; charset=UTF-8 ...
## [2] <body jsmodel="hspDDf">\n<header id="hdr"><script nonce="MtcCYcFM7I2FO2eU ...
vals <- list(q = "web scraping", hl = "fr")
search <- search |> html_form_set(!!!vals)
resp <- html_form_submit(search)
read_html(resp)
## {html_document}
## <html lang="fr">
## [1] <head>\n<meta http-equiv="Content-Type" content="text/html; charset=UTF-8 ...
## [2] <body jsmodel="hspDDf">\n<header id="hdr"><script nonce="XgWmDZMPbs6Meu4A ...
If you are working with a session, the workflow is as follows:
- Extract the form.
- Set it.
- Start your session on the page with the form.
- Submit the form using
session_submit()
.
google_form <- read_html("http://www.google.com") |>
html_form() |>
pluck(1) #another way to do [[1]]
search_something <- google_form |> html_form_set(q = "something")
google_session <- session("http://www.google.com") |>
session_submit(search_something, submit = "btnG")
google_session |>
read_html()
## {html_document}
## <html lang="fr">
## [1] <head>\n<meta http-equiv="Content-Type" content="text/html; charset=UTF-8 ...
## [2] <body jsmodel="hspDDf">\n<header id="hdr"><script nonce="z5XlV809ZwAsSx_K ...
2.1.4 Scraping hacks
Some web pages are a bit fancier than the ones we have looked at so far (i.e., they use JavaScript). rvest
works nicely for static web pages, but for more advanced ones you need different tools such as RSelenium
. This, however, goes beyond the scope of this tutorial.
A web page may sometimes give you time-outs (i.e., it doesn’t respond within a given time). This can break your loop. Wrapping your code in safely()
or insistently()
from the purrr
package might help. The former moves on and notes down what has gone wrong, the latter keeps sending requests until it has been successful. They both work easiest if you put your scraping code in functions and wrap those with either insistently()
or safely()
.
Sometimes a web page keeps blocking you. Consider using a proxy server.
my_proxy <- httr::use_proxy(url = "http://example.com",
user_name = "myusername",
password = "mypassword",
auth = "one of basic, digest, digest_ie, gssnegotiate, ntlm, any")
my_session <- session("https://scrapethissite.com/", my_proxy)
Find more useful information – including the stuff we just described – and links on this GitHub page.
2.2 Application Programming Interfaces (APIs)
While web scraping (or screen scraping, as you extract the stuff that appears on your screen) is certainly fun, it should be seen as a last resort. More and more web platforms provide so-called Application Programming Interfaces (APIs).
“An application programming interface (API) is a connection between computers or between computer programs.” (Wikipedia)
There are a bunch of different sorts of APIs, but the most common one is the REST API. REST stands for “REpresentational State Transfer” and describes a set of rules the API designers are supposed to obey when developing their particular interface. You can make different requests, such as GET content, POST a file to a server – PUT
is similar, or request to DELETE
a file. We will only focus on the GET
part.
APIs offer you a structured way to communicate with the platform via your machine. In our use case, this means that you can get the data you want in a usually well-structured format and without all the “dirt” that you need to scrape off tediously (enough web scraping metaphors for today). With APIs, you can generally quite clearly define what you want and how you want it. In R, we achieve this by using the httr
(Wickham 2020) package. Moreover, using APIs does not bear the risk of acquiring the information you are not supposed to access and you also do not need to worry about the server not being able to handle the load of your requests (usually, there are rate limits in place to address this particular issue). However, it’s not all fun and games with APIs: they might give you their data in a special format, both XML and JSON are common. The former is the one rvest
uses as well, the latter can be tamed using jsonlite
(Ooms, Temple Lang, and Hilaiel 2020) which is to be introduced as well. Moreover, you usually have to ask the platform for permission and perhaps pay to get it. Once you have received the keys you need, you can tell R to fill them automatically, similar to how your browser knows your Amazon password, etc.; usethis
(Wickham et al. 2021) can help you with such tasks.
The best thing that can happen with APIs: some of them are so popular that people have already written specific R packages for working with them – an overview can be found on the ROpenSci website. One example of this was Twitter and the rtweet
package (Kearney 2019).
2.2.1 Obtaining their data
API requests are performed using URLs. Those start with the basic address of the API (e.g., https://api.nytimes.com), followed by the endpoint that you want to use (e.g., /lists). They also contain so-called headers which are provided as key-value pairs. Those headers can contain for instance authentication tokens or different search parameters. A request to the New York Times API to obtain articles for January 2019 would then look like this: https://api.nytimes.com/svc/archive/v1/2019/1.json?api-key=yourkey.
At most APIs, you will have to register first. As we will play with the New York Times API, do this here.
2.2.2 Making queries
A basic query is performed using the GET()
function. However, first, you need to define the call you want to make. The different keys and values they can take can be found in the API documentation. Of course, there is also a neater way to deal with the key problem. We will show it later.
needs(httr, jsonlite)
#see overview here: https://developer.nytimes.com/docs/timeswire-product/1/overview
key <- "xxx"
#key <- Sys.getenv("nyt_key")
nyt_headlines <- modify_url(
url = "https://api.nytimes.com/",
path = "svc/news/v3/content/nyt/business.json",
query = list(`api-key` = key))
response <- GET(nyt_headlines)
When it comes to the NYT news API, there is the problem that the type of section is specified not in the query but in the endpoint path itself. Hence, if we were to scrape the different sections, we would have to change the path itself, e.g., through str_c()
.
paths <- str_c("svc/news/v3/content/nyt/", c("business", "world"), ".json")
map(paths,
\(x) GET(modify_url(
url = "https://api.nytimes.com/",
path = x,
query = list(`api-key` = key))
)
)
## [[1]]
## Response [https://api.nytimes.com/svc/news/v3/content/nyt/business.json?api-key=xxx]
## Date: 2023-06-26 16:09
## Status: 401
## Content-Type: application/json
## Size: 90 B
##
##
## [[2]]
## Response [https://api.nytimes.com/svc/news/v3/content/nyt/world.json?api-key=xxx]
## Date: 2023-06-26 16:09
## Status: 401
## Content-Type: application/json
## Size: 90 B
The Status:
code you want to see here is 200
which stands for success. If you want to put it inside a function, you might want to break the function once you get a non-successful query. http_error()
or http_status()
are your friends here.
## [1] TRUE
## $category
## [1] "Client error"
##
## $reason
## [1] "Unauthorized"
##
## $message
## [1] "Client error: (401) Unauthorized"
content()
will give you the content of the request.
What you see is also the content of the call – which is what we want. It is in a format that we cannot work with right away, though, it is in JSON.
2.2.3 JSON
The following unordered list is stolen from this blog entry:
- The data are in name/value pairs
- Commas separate data objects
- Curly brackets {} hold objects
- Square brackets [] hold arrays
- Each data element is enclosed with quotes “” if it is a character, or without quotes if it is a numeric value
jsonlite
helps us to bring this output into a data frame.
## $fault
## $fault$faultstring
## [1] "Invalid ApiKey"
##
## $fault$detail
## $fault$detail$errorcode
## [1] "oauth.v2.InvalidApiKey"
2.2.4 Dealing with authentification
Well, as we saw before, we would have to put our official NYT API key publicly visible in this script. This is bad practice and should be avoided, especially if you work on a joint project (where everybody uses their code) or if you put your scripts in public places (such as GitHub). The usethis
package can help you here.
2.3 Exercises
- Read in the Start a session with the tidyverse Wikipedia page. Adapt your user agent to some sort of different value. Proceed to Hadley Wickham’s page. Go back. Go forth. Jump to a different Wikipedia page. Check the
session_history()
to see if it has worked.
tidyverse_wiki <- "https://en.wikipedia.org/wiki/Tidyverse"
hadley_wiki <- "https://en.wikipedia.org/wiki/Hadley_Wickham"
etienne_wiki <- "https://fr.wikipedia.org/wiki/Étienne_Ollion"
user_agent <- user_agent("Hi, I'm Felix and I'm trying to steal your data.")
wiki_session <- session(tidyverse_wiki, user_agent)
wiki_session_jumpled <- wiki_session |>
session_jump_to(hadley_wiki) |>
session_back() |>
session_forward() |>
session_jump_to(etienne_wiki)
wiki_session |> session_history()
- Start a session on “https://www.scrapethissite.com/pages/advanced/?gotcha=login”, fill out, and submit the form. Any value for login and password will do. (Disclaimer: you have to add the URL as an “action” attribute after creating the form, see this thread. – so after extracting and setting the form, include and run
login_form$action <- url
) before submitting it.
url <- "https://www.scrapethissite.com/pages/advanced/?gotcha=login"
#extract and set login form here
login_form$action <- url # add url as action attribute
# submit form
base_session <- session("https://www.scrapethissite.com/pages/advanced/?gotcha=login") |>
session_submit(login_form)
url <- "https://www.scrapethissite.com/pages/advanced/?gotcha=login"
#extract and set login form here
login_form <- read_html(url) |>
html_form() |>
pluck(1) |>
html_form_set(user = "123",
pass = "456")
login_form$action <- url # add url as action attribute
# submit form
base_session <- session("https://www.scrapethissite.com/pages/advanced/?gotcha=login") %>%
session_submit(login_form)
base_session |> read_html() |> html_text()
- Search for articles on the NYT API (find the proper parameters here) that deal with a certain topic (parameter “q”), set a certain begin and end date. Extract the results into a tibble.
Bonus: Provide the key by using the Sys.getenv
function. So, if somebody wants to work with your code and their own key, all they need to make sure is that they have the API key stored in the environment with the same name.
trump_nov_2016 <- modify_url(
url = "http://api.nytimes.com/svc/search/v2/articlesearch.json",
query = list(q = "Trump",
begin_date = "20161101",
end_date = "20161110",
`api-key` = Sys.getenv("nyt_key"))
) |>
GET()
trump_nov_2016 |>
content(as = "text") |>
fromJSON()
## No encoding supplied: defaulting to UTF-8.
## $status
## [1] "OK"
##
## $copyright
## [1] "Copyright (c) 2023 The New York Times Company. All Rights Reserved."
##
## $response
## $response$docs
## abstract
## 1 Mr. Trump upended the G.O.P. and now the Democratic Party, and America’s fierce and heedless desire for change now puts the nation on a precipice.
## 2 There are crucial lessons in the 2016 race’s cruel turn.
## 3 From the economy to relations with Russia and everything in between, the new president could spell big trouble for the Continent.
## 4 Donald J. Trump voted at a polling place in Manhattan, just blocks from his home and office at Trump Tower.
## 5 He had an intuition about American anger. The country’s liberal elites were too arrogant to take him seriously.
## 6 With G.O.P. majorities in Congress, his authority is great.
## 7 His surprise victory ended an erratic and grievance-filled campaign that took direct aim at his own party and long-held ideals of American democracy.
## 8 Republicans are indicating that if they can’t gain control of government fairly, they’ll simply undermine it.
## 9 The Republican candidate and the deceased Venezuelan president are both populists by posture, if not ideology.
## 10 If the G.O.P. loses the presidential election, its reconstruction must begin Nov. 9. If it wins, we have to start over.
## web_url
## 1 https://www.nytimes.com/2016/11/09/opinion/donald-trumps-revolt.html
## 2 https://www.nytimes.com/2016/11/09/opinion/donald-trumps-shocking-success.html
## 3 https://www.nytimes.com/2016/11/11/opinion/for-europe-trumps-election-is-a-terrifying-disaster.html
## 4 https://www.nytimes.com/video/us/politics/100000004755887/donald-trump-votes.html
## 5 https://www.nytimes.com/2016/11/10/opinion/president-donald-trump.html
## 6 https://www.nytimes.com/2016/11/10/opinion/are-there-limits-to-trumps-power.html
## 7 https://www.nytimes.com/2016/11/09/us/politics/hillary-clinton-donald-trump-president.html
## 8 https://www.nytimes.com/2016/11/04/opinion/donald-trumps-impeachment-threat.html
## 9 https://www.nytimes.com/2016/11/04/opinion/is-donald-trump-an-american-hugo-chavez.html
## 10 https://www.nytimes.com/2016/11/06/opinion/campaign-stops/is-there-life-after-trump.html
## snippet
## 1 Mr. Trump upended the G.O.P. and now the Democratic Party, and America’s fierce and heedless desire for change now puts the nation on a precipice.
## 2 There are crucial lessons in the 2016 race’s cruel turn.
## 3 From the economy to relations with Russia and everything in between, the new president could spell big trouble for the Continent.
## 4 Donald J. Trump voted at a polling place in Manhattan, just blocks from his home and office at Trump Tower.
## 5 He had an intuition about American anger. The country’s liberal elites were too arrogant to take him seriously.
## 6 With G.O.P. majorities in Congress, his authority is great.
## 7 His surprise victory ended an erratic and grievance-filled campaign that took direct aim at his own party and long-held ideals of American democracy.
## 8 Republicans are indicating that if they can’t gain control of government fairly, they’ll simply undermine it.
## 9 The Republican candidate and the deceased Venezuelan president are both populists by posture, if not ideology.
## 10 If the G.O.P. loses the presidential election, its reconstruction must begin Nov. 9. If it wins, we have to start over.
## lead_paragraph
## 1 President Donald Trump. Three words that were unthinkable to tens of millions of Americans — and much of the rest of the world — have now become the future of the United States.
## 2 Just days ago I was in Ohio. I was talking to Republicans, and this was the refrain I kept hearing: Donald Trump is throwing this election away. He has no real campaign here. No get-out-the-vote operation. No ground game. Nothing that signifies or befits a truly serious presidential candidate.
## 3 WASHINGTON — No one in Europe truly believed Americans would elect someone who seems so obviously unfit to lead the most powerful nation in the world. And yet, that is precisely what has happened, and now, across the Continent, people are trying to figure out what this will mean. Many fear that Donald J. Trump’s election might mean the end of the West as we know it.
## 4 Donald J. Trump voted at a polling place in Manhattan, just blocks from his home and office at Trump Tower.
## 5 President Donald Trump. Get used to it. The world as we knew it is no more.
## 6 When Donald J. Trump takes office on Jan. 20, he will have immense but not unlimited power at his disposal. Back in June, I wrote that he could — even without congressional consent — execute much of his vision. He could, for example, shift enforcement priorities against unauthorized immigrants, probably bar Muslims from entering the country, raise tariffs, withdraw forces from the Middle East, and tear up trade treaties and military alliances.
## 7 Donald John Trump was elected the 45th president of the United States on Tuesday in a stunning culmination of an explosive, populist and polarizing campaign that took relentless aim at the institutions and long-held ideals of American democracy.
## 8 Donald Trump and other embattled Republican candidates are resorting to a particularly bizarre and dangerous tactic in the closing days of the campaign — warning that they may well seek to impeach Hillary Clinton if she wins, or, short of that, tie her up with endless investigations and other delaying tactics.
## 9 MEXICO CITY — To dramatic music, the video starts with a clip of the Republican nominee for president, Donald J. Trump, threatening to jail his rival, Hillary Clinton, before the words “Te recuerda a alguien” (“Does this remind you of someone”) pop up. The image switches to the former Venezuelan president Hugo Chávez, founder of the self-declared Socialist revolution, who steered his oil-rich nation to meltdown.
## 10 With only hours left before Election Day, here’s the painful, self-evident truth facing lifelong Republicans like me: The 2016 presidential campaign has revealed dark and disturbing things about not only Donald J. Trump but also the party that nominated him.
## print_section print_page source
## 1 A 26 The New York Times
## 2 A 27 The New York Times
## 3 A 15 The New York Times
## 4 <NA> <NA> <NA>
## 5 A 14 The New York Times
## 6 <NA> <NA> The New York Times
## 7 A 1 The New York Times
## 8 A 28 The New York Times
## 9 A 17 The New York Times
## 10 SR 5 The New York Times
## multimedia
## 1 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/10/opinion/09wed4web/09wed4web-sfSpan.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-largeHorizontalJumbo.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-largeHorizontal375.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoFifteenBySeven1305.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoFifteenBySeven2610.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-miniMoth.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-windowsTile336H.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-facebookJumbo.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-hpLarge.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-largeWidescreen573.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-largeWidescreen1050.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-thumbStandard-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-thumbLarge-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-smallSquare252-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blogSmallThumb-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-smallSquare168-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-thumbWide.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoThumb.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoLarge.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumThreeByTwo210.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumThreeByTwo225.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumThreeByTwo440.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumThreeByTwo252.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumThreeByTwo378.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSmall.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoHpMedium.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine600.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine540.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine495.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine390.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine480.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine310.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine225.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine96.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine768.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine150.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine1050.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNine3000.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-videoSixteenByNineJumbo1600.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-square320.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-filmstrip.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-square640.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-moth.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumSquare149.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-articleInline.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-hpSmall.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blogSmallInline.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-mediumFlexible177.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-watch308-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-watch268-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-verticalTwoByThree735-v2.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-articleLarge.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blog480.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blog427.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-tmagArticle.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-jumbo.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blog225.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master675.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master180.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master768.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-popup.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-blog533.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-tmagSF.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-slide.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-superJumbo.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master1050.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master495.jpg, images/2016/11/10/opinion/09wed4web/09wed4web-master315.jpg, 263, 683, 250, 609, 1218, 70, 336, 550, 288, 322, 591, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1687, 900, 320, 190, 640, 151, 149, 127, 109, 101, 118, 348, 303, 1103, 400, 320, 285, 395, 683, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 395, 1024, 375, 1305, 2610, 151, 694, 1050, 511, 573, 1050, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, 320, 190, 640, 151, 149, 190, 163, 151, 177, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09wed4web/09wed4web-thumbStandard-v2.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09wed4web/09wed4web-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09wed4web/09wed4web-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315
## 2 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, facebookJumbo, verticalTwoByThree735, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, sfSpan, largeHorizontalJumbo, largeHorizontal375, articleInline, hpSmall, blogSmallInline, mediumFlexible177, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, watch308, watch268, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-facebookJumbo.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-verticalTwoByThree735.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-hpLarge.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-largeWidescreen573.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-largeWidescreen1050.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-articleLarge.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blog480.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blog427.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-tmagArticle.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-jumbo.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blog225.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master675.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master180.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master768.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-popup.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blog533.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-tmagSF.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-slide.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-superJumbo.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master1050.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master495.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-master315.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoFifteenBySeven1305.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoFifteenBySeven2610.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-miniMoth.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-windowsTile336H.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-sfSpan.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-largeHorizontalJumbo.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-largeHorizontal375.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-articleInline.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-hpSmall.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blogSmallInline.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumFlexible177.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-thumbWide.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoThumb.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoLarge.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumThreeByTwo210.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumThreeByTwo225.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumThreeByTwo440.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumThreeByTwo252.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumThreeByTwo378.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-thumbStandard-v2.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-thumbLarge-v2.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-smallSquare252-v2.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-blogSmallThumb-v2.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-smallSquare168-v2.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-watch308.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-watch268.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSmall.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoHpMedium.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine600.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine540.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine495.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine390.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine480.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine310.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine225.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine96.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine768.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine150.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNine1050.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-videoSixteenByNineJumbo1600.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-square320.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-filmstrip.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-square640.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-moth.jpg, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-mediumSquare149.jpg, 550, 1102, 287, 322, 590, 372, 297, 264, 367, 634, 139, 418, 111, 476, 403, 330, 224, 372, 1268, 650, 307, 195, 609, 1218, 70, 336, 263, 683, 250, 118, 101, 94, 110, 126, 50, 507, 140, 150, 293, 168, 252, 75, 150, 252, 50, 168, 348, 303, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 900, 320, 190, 640, 151, 149, 1050, 735, 511, 573, 1050, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 1305, 2610, 151, 694, 395, 1024, 375, 190, 163, 151, 177, 190, 75, 768, 210, 225, 440, 252, 378, 75, 150, 252, 50, 168, 312, 272, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 1600, 320, 190, 640, 151, 149, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 372, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/opinion/09bruniWeb/09bruniWeb-thumbStandard-v2.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, facebookJumbo, verticalTwoByThree735, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, sfSpan, largeHorizontalJumbo, largeHorizontal375, articleInline, hpSmall, blogSmallInline, mediumFlexible177, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, watch308, watch268, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, facebookJumbo, verticalTwoByThree735, hpLarge, largeWidescreen573, largeWidescreen1050, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, sfSpan, largeHorizontalJumbo, largeHorizontal375, articleInline, hpSmall, blogSmallInline, mediumFlexible177, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, watch308, watch268, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149
## 3 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoFifteenBySeven1305.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoFifteenBySeven2610.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-miniMoth.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-windowsTile336H.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-watch308.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-watch268.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-verticalTwoByThree735.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-articleLarge.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blog480.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blog427.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-tmagArticle.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-jumbo.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blog225.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master675.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master180.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master768.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-popup.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blog533.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-tmagSF.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-slide.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-superJumbo.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master1050.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master495.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-master315.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-square320.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-filmstrip.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-square640.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-moth.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumSquare149.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-sfSpan.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-largeHorizontalJumbo.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-largeHorizontal375.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-thumbStandard.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-thumbLarge.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-smallSquare252.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blogSmallThumb.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-smallSquare168.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-thumbWide.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoThumb.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoLarge.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumThreeByTwo210.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumThreeByTwo225.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumThreeByTwo440.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumThreeByTwo252.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumThreeByTwo378.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-articleInline.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-hpSmall.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-blogSmallInline.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-mediumFlexible177.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-facebookJumbo.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-hpLarge.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-largeWidescreen573.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-largeWidescreen1050.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSmall.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoHpMedium.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine600.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine540.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine495.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine390.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine480.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine310.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine225.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine96.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine768.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine150.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine1050.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNine3000.jpg, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-videoSixteenByNineJumbo1600.jpg, 609, 1218, 70, 336, 348, 304, 1103, 454, 364, 323, 448, 776, 170, 511, 136, 582, 492, 404, 274, 454, 1551, 795, 375, 239, 320, 190, 640, 151, 149, 264, 683, 250, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 144, 123, 114, 134, 550, 288, 322, 591, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1688, 900, 1305, 2610, 151, 694, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 320, 190, 640, 151, 149, 395, 1024, 375, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 190, 163, 151, 177, 1050, 511, 573, 1050, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 454, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/11wergin-inyt/00wergin-inyt-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600
## 4 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSmall.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoHpMedium.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine600.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine540.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine495.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine390.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine480.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine310.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine225.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine96.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine768.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine150.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine1050.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNine3000.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoSixteenByNineJumbo1600.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-sfSpan.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-largeHorizontalJumbo.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-largeHorizontal375.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-square320.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-filmstrip.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-square640.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-moth.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumSquare149.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-watch308.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-watch268.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-facebookJumbo.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-thumbWide.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoThumb.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoLarge.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumThreeByTwo210.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumThreeByTwo225.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumThreeByTwo440.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumThreeByTwo252.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumThreeByTwo378.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-thumbStandard.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-thumbLarge.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-smallSquare252.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blogSmallThumb.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-smallSquare168.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-hpLarge.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-largeWidescreen573.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-largeWidescreen1050.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-articleLarge.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blog480.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blog427.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-tmagArticle.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-jumbo.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blog225.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master675.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master180.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master768.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-popup.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blog533.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-tmagSF.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-slide.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-superJumbo.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master1050.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master495.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-master315.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-verticalTwoByThree735.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-articleInline.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-hpSmall.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-blogSmallInline.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-mediumFlexible177.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoFifteenBySeven1305.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-videoFifteenBySeven2610.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-miniMoth.jpg, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-windowsTile336H.jpg, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1687, 900, 263, 683, 250, 320, 190, 640, 151, 149, 348, 303, 550, 126, 50, 507, 140, 150, 293, 168, 252, 75, 150, 252, 50, 168, 287, 322, 590, 400, 320, 285, 395, 683, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 1103, 127, 109, 101, 118, 609, 1218, 70, 336, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, 395, 1024, 375, 320, 190, 640, 151, 149, 312, 272, 1050, 190, 75, 768, 210, 225, 440, 252, 378, 75, 150, 252, 50, 168, 511, 573, 1050, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 735, 190, 163, 151, 177, 1305, 2610, 151, 694, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/09/us/09fd-trumpvotes3/09fd-trumpvotes3-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H
## 5 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-sfSpan.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-largeHorizontalJumbo.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-largeHorizontal375.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoFifteenBySeven1305.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoFifteenBySeven2610.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-miniMoth.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-windowsTile336H.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-facebookJumbo.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-hpLarge.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-largeWidescreen573.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-largeWidescreen1050.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-thumbStandard.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-thumbLarge.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-smallSquare252.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blogSmallThumb.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-smallSquare168.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-thumbWide.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoThumb.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoLarge.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumThreeByTwo210.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumThreeByTwo225.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumThreeByTwo440.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumThreeByTwo252.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumThreeByTwo378.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSmall.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoHpMedium.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine600.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine540.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine495.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine390.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine480.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine310.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine225.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine96.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine768.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine150.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine1050.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNine3000.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-videoSixteenByNineJumbo1600.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-square320.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-filmstrip.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-square640.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-moth.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumSquare149.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-articleInline.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-hpSmall.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blogSmallInline.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-mediumFlexible177.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-watch308.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-watch268.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-verticalTwoByThree735.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-articleLarge.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blog480.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blog427.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-tmagArticle.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-jumbo.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blog225.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master675.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master180.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master768.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-popup.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-blog533.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-tmagSF.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-slide.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-superJumbo.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master1050.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master495.jpg, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-master315.jpg, 263, 683, 250, 609, 1218, 70, 336, 550, 287, 322, 590, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1687, 900, 320, 190, 640, 151, 149, 127, 109, 101, 118, 348, 303, 1102, 400, 320, 285, 395, 683, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 395, 1024, 375, 1305, 2610, 151, 694, 1050, 511, 573, 1050, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, 320, 190, 640, 151, 149, 190, 163, 151, 177, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/world/10COHEN-inyt/10COHEN-inyt-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315
## 6 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoFifteenBySeven1305.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoFifteenBySeven2610.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-miniMoth.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-windowsTile336H.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-watch308.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-watch268.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-verticalTwoByThree735.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-articleLarge.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blog480.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blog427.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-tmagArticle.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-jumbo.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blog225.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master675.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master180.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master768.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-popup.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blog533.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-tmagSF.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-slide.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-superJumbo.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master1050.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master495.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-master315.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-square320.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-filmstrip.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-square640.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-moth.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumSquare149.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-sfSpan.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-largeHorizontalJumbo.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-largeHorizontal375.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-thumbStandard.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-thumbLarge.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-smallSquare252.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blogSmallThumb.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-smallSquare168.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-thumbWide.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoThumb.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoLarge.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumThreeByTwo210.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumThreeByTwo225.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumThreeByTwo440.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumThreeByTwo252.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumThreeByTwo378.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-articleInline.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-hpSmall.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-blogSmallInline.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-mediumFlexible177.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-facebookJumbo.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-hpLarge.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-largeWidescreen573.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-largeWidescreen1050.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSmall.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoHpMedium.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine600.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine540.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine495.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine390.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine480.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine310.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine225.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine96.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine768.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine150.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNine1050.jpg, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-videoSixteenByNineJumbo1600.jpg, 609, 1218, 70, 336, 348, 303, 1102, 400, 320, 285, 395, 683, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 320, 190, 640, 151, 149, 263, 683, 250, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 127, 109, 101, 118, 550, 287, 322, 591, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 899, 1305, 2610, 151, 694, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 320, 190, 640, 151, 149, 395, 1024, 375, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 190, 163, 151, 177, 1050, 511, 573, 1050, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 1600, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/11/opinion/10posnerWeb/10posnerWeb-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600
## 7 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/10/us/10electweb1/10electweb1-sfSpan-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-largeHorizontalJumbo-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-largeHorizontal375-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoFifteenBySeven1305-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoFifteenBySeven2610-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-miniMoth-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-windowsTile336H-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-facebookJumbo-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-hpLarge-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-largeWidescreen573-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-largeWidescreen1050-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-thumbStandard-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-thumbLarge-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-smallSquare252-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-blogSmallThumb-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-smallSquare168-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-thumbWide-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoThumb-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoLarge-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumThreeByTwo210-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumThreeByTwo225-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumThreeByTwo440-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumThreeByTwo252-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumThreeByTwo378-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSmall-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoHpMedium-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine600-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine540-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine495-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine390-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine480-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine310-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine225-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine96-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine768-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine150-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine1050-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNine3000-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-videoSixteenByNineJumbo1600-v4.jpg, images/2016/11/10/us/10electweb1/10electweb1-square320-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-filmstrip-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-square640-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-moth-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumSquare149-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-articleInline-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-hpSmall-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-blogSmallInline-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-mediumFlexible177-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-watch308-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-watch268-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-verticalTwoByThree735-v3.jpg, images/2016/11/10/us/10electweb1/10electweb1-articleLarge-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-blog480-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-blog427-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-tmagArticle-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-jumbo-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-blog225-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master675-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master180-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master768-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-popup-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-blog533-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-tmagSF-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-slide-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-superJumbo-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master1050-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master495-v2.jpg, images/2016/11/10/us/10electweb1/10electweb1-master315-v2.jpg, 263, 683, 250, 609, 1218, 70, 336, 550, 287, 322, 590, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1687, 900, 320, 190, 640, 151, 149, 127, 109, 101, 118, 348, 303, 1102, 400, 320, 285, 394, 682, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 395, 1024, 375, 1305, 2610, 151, 694, 1050, 511, 573, 1050, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, 320, 190, 640, 151, 149, 190, 163, 151, 177, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/us/10electweb1/10electweb1-thumbStandard-v4.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/us/10electweb1/10electweb1-thumbWide-v2.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/10/us/10electweb1/10electweb1-articleLarge-v2.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, sfSpan, largeHorizontalJumbo, largeHorizontal375, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, square320, filmstrip, square640, moth, mediumSquare149, articleInline, hpSmall, blogSmallInline, mediumFlexible177, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315
## 8 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/04/opinion/04fri1web/04fri1web-videoFifteenBySeven1305.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoFifteenBySeven2610.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-miniMoth.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-windowsTile336H.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-watch308.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-watch268.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-verticalTwoByThree735.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-articleLarge.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blog480.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blog427.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-tmagArticle.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-jumbo.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blog225.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master675.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master180.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master768.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-popup.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blog533.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-tmagSF.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-slide.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-superJumbo.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master1050.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master495.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-master315.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-square320.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-filmstrip.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-square640.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-moth.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumSquare149.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-sfSpan.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-largeHorizontalJumbo.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-largeHorizontal375.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-thumbStandard.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-thumbLarge.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-smallSquare252.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blogSmallThumb.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-smallSquare168.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-thumbWide.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoThumb.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoLarge.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumThreeByTwo210.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumThreeByTwo225.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumThreeByTwo440.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumThreeByTwo252.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumThreeByTwo378.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-articleInline.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-hpSmall.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-blogSmallInline.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-mediumFlexible177.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-facebookJumbo.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-hpLarge.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-largeWidescreen573.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-largeWidescreen1050.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSmall.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoHpMedium.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine600.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine540.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine495.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine390.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine480.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine310.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine225.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine96.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine768.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine150.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine1050.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNine3000.jpg, images/2016/11/04/opinion/04fri1web/04fri1web-videoSixteenByNineJumbo1600.jpg, 609, 1218, 70, 336, 348, 303, 1103, 400, 320, 285, 395, 683, 150, 450, 120, 512, 433, 355, 241, 400, 1365, 700, 330, 210, 320, 190, 640, 151, 149, 263, 683, 250, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 127, 109, 101, 118, 550, 287, 322, 590, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 1688, 900, 1305, 2610, 151, 694, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 320, 190, 640, 151, 149, 395, 1024, 375, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 190, 163, 151, 177, 1050, 511, 573, 1050, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 3000, 1600, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04fri1web/04fri1web-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 400, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04fri1web/04fri1web-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04fri1web/04fri1web-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNine3000, videoSixteenByNineJumbo1600
## 9 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoFifteenBySeven1305.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoFifteenBySeven2610.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-miniMoth.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-windowsTile336H.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-watch308.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-watch268.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-verticalTwoByThree735.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-articleLarge.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blog480.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blog427.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-tmagArticle.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-jumbo.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blog225.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master675.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master180.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master768.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-popup.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blog533.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-tmagSF.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-slide.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-superJumbo.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master1050.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master495.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-master315.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-square320.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-filmstrip.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-square640.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-moth.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumSquare149.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-sfSpan.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-largeHorizontalJumbo.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-largeHorizontal375.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-thumbStandard.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-thumbLarge.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-smallSquare252.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blogSmallThumb.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-smallSquare168.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-thumbWide.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoThumb.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoLarge.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumThreeByTwo210.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumThreeByTwo225.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumThreeByTwo440.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumThreeByTwo252.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumThreeByTwo378.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-articleInline.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-hpSmall.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-blogSmallInline.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-mediumFlexible177.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-facebookJumbo.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-hpLarge.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-largeWidescreen573.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-largeWidescreen1050.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSmall.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoHpMedium.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine600.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine540.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine495.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine390.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine480.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine310.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine225.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine96.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine768.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine150.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNine1050.jpg, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-videoSixteenByNineJumbo1600.jpg, 609, 1218, 70, 336, 348, 304, 1102, 283, 227, 202, 280, 484, 106, 319, 85, 363, 307, 252, 171, 283, 967, 496, 234, 149, 320, 190, 640, 151, 149, 263, 683, 250, 75, 150, 252, 50, 168, 126, 50, 507, 140, 150, 293, 168, 252, 90, 77, 71, 84, 550, 288, 322, 591, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 900, 1305, 2610, 151, 694, 312, 272, 735, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 320, 190, 640, 151, 149, 395, 1024, 375, 75, 150, 252, 50, 168, 190, 75, 768, 210, 225, 440, 252, 378, 190, 163, 151, 177, 1050, 511, 573, 1050, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 1600, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 283, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/04/opinion/04GRILLO-inyt/04GRILLO-inyt-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, watch308, watch268, verticalTwoByThree735, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, square320, filmstrip, square640, moth, mediumSquare149, sfSpan, largeHorizontalJumbo, largeHorizontal375, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, articleInline, hpSmall, blogSmallInline, mediumFlexible177, facebookJumbo, hpLarge, largeWidescreen573, largeWidescreen1050, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600
## 10 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, image, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSmall.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoHpMedium.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine600.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine540.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine495.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine390.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine480.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine310.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine225.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine96.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine768.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine150.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNine1050.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoSixteenByNineJumbo1600.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-sfSpan.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-largeHorizontalJumbo.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-largeHorizontal375.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-square320.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-filmstrip.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-square640.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-moth.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumSquare149.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-watch308.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-watch268.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-facebookJumbo.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-thumbWide.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoThumb.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoLarge.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumThreeByTwo210.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumThreeByTwo225.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumThreeByTwo440.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumThreeByTwo252.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumThreeByTwo378.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-thumbStandard.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-thumbLarge.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-smallSquare252.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blogSmallThumb.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-smallSquare168.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-hpLarge.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-largeWidescreen573.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-largeWidescreen1050.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-articleLarge.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blog480.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blog427.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-tmagArticle.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-jumbo.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blog225.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master675.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master180.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master768.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-popup.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blog533.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-tmagSF.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-slide.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-superJumbo.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master1050.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master495.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-master315.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-verticalTwoByThree735.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-articleInline.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-hpSmall.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-blogSmallInline.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-mediumFlexible177.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoFifteenBySeven1305.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-videoFifteenBySeven2610.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-miniMoth.jpg, images/2016/11/06/opinion/sunday/06wehner/06wehner-windowsTile336H.jpg, 281, 211, 338, 304, 278, 219, 270, 174, 126, 54, 432, 84, 591, 900, 263, 683, 250, 320, 190, 640, 151, 149, 348, 303, 549, 126, 50, 507, 140, 150, 293, 168, 252, 75, 150, 252, 50, 168, 287, 322, 591, 403, 322, 287, 398, 688, 151, 453, 121, 516, 437, 358, 243, 403, 1376, 705, 333, 212, 1102, 128, 110, 101, 119, 609, 1218, 70, 336, 500, 375, 600, 540, 495, 390, 480, 310, 225, 96, 768, 150, 1050, 1600, 395, 1024, 375, 320, 190, 640, 151, 149, 312, 272, 1050, 190, 75, 768, 210, 225, 440, 252, 378, 75, 150, 252, 50, 168, 511, 573, 1050, 600, 480, 427, 592, 1024, 225, 675, 180, 768, 650, 533, 362, 600, 2048, 1050, 495, 315, 735, 190, 163, 151, 177, 1305, 2610, 151, 694, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 190, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 126, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/06/opinion/sunday/06wehner/06wehner-thumbWide.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/06/opinion/sunday/06wehner/06wehner-thumbStandard.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 75, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, images/2016/11/06/opinion/sunday/06wehner/06wehner-articleLarge.jpg, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 600, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, 403, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, wide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbnail, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, xlarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H, videoSmall, videoHpMedium, videoSixteenByNine600, videoSixteenByNine540, videoSixteenByNine495, videoSixteenByNine390, videoSixteenByNine480, videoSixteenByNine310, videoSixteenByNine225, videoSixteenByNine96, videoSixteenByNine768, videoSixteenByNine150, videoSixteenByNine1050, videoSixteenByNineJumbo1600, sfSpan, largeHorizontalJumbo, largeHorizontal375, square320, filmstrip, square640, moth, mediumSquare149, watch308, watch268, facebookJumbo, thumbWide, videoThumb, videoLarge, mediumThreeByTwo210, mediumThreeByTwo225, mediumThreeByTwo440, mediumThreeByTwo252, mediumThreeByTwo378, thumbStandard, thumbLarge, smallSquare252, blogSmallThumb, smallSquare168, hpLarge, largeWidescreen573, largeWidescreen1050, articleLarge, blog480, blog427, tmagArticle, jumbo, blog225, master675, master180, master768, popup, blog533, tmagSF, slide, superJumbo, master1050, master495, master315, verticalTwoByThree735, articleInline, hpSmall, blogSmallInline, mediumFlexible177, videoFifteenBySeven1305, videoFifteenBySeven2610, miniMoth, windowsTile336H
## headline.main
## 1 Donald Trump’s Revolt
## 2 Donald Trump’s Shocking Success
## 3 For Europe, Trump’s Election Is a Terrifying Disaster
## 4 Donald Trump Votes
## 5 President Donald Trump
## 6 Are There Limits to Trump’s Power?
## 7 Donald Trump Is Elected President in Stunning Repudiation of the Establishment
## 8 Donald Trump’s Impeachment Threat
## 9 Is Donald Trump an American Hugo Chávez?
## 10 Is There Life After Trump?
## headline.kicker headline.content_kicker
## 1 Editorial NA
## 2 Op-Ed Columnist NA
## 3 Op-Ed Contributor NA
## 4 <NA> NA
## 5 Op-Ed Columnist NA
## 6 Op-Ed Contributor NA
## 7 <NA> NA
## 8 Editorial NA
## 9 Contributing Op-Ed Writer NA
## 10 Contributing Op-Ed Writer NA
## headline.print_headline headline.name headline.seo headline.sub
## 1 The Trump Revolt NA NA NA
## 2 Trump’s Alarming Success NA NA NA
## 3 Trump: A disaster for Europe? NA NA NA
## 4 <NA> NA NA NA
## 5 President Donald Trump NA NA NA
## 6 <NA> NA NA NA
## 7 Trump Triumphs NA NA NA
## 8 Mr. Trump’s Impeachment Threat NA NA NA
## 9 Is Trump the U.S. Chávez? NA NA NA
## 10 Is There Life After Trump? NA NA NA
## keywords
## 1 subject, persons, organizations, persons, subject, Presidential Election of 2016, Trump, Donald J, Republican Party, Clinton, Hillary Rodham, Editorials, 1, 2, 3, 4, 5, N, N, N, N, N
## 2 subject, persons, organizations, persons, subject, Presidential Election of 2016, Trump, Donald J, Republican Party, Clinton, Hillary Rodham, Polls and Public Opinion, 1, 3, 4, 5, 6, N, N, N, N, N
## 3 subject, subject, organizations, organizations, persons, persons, persons, glocations, Presidential Election of 2016, International Trade and World Market, European Union, North Atlantic Treaty Organization, Merkel, Angela, Putin, Vladimir V, Trump, Donald J, Europe, 1, 2, 3, 4, 5, 6, 7, 8, N, N, N, N, N, N, N, N
## 4 persons, glocations, subject, Trump, Donald J, Manhattan (NYC), Voting and Voters, 1, 2, 3, N, N, N
## 5 persons, subject, Trump, Donald J, Presidential Election of 2016, 1, 2, N, N
## 6 subject, subject, subject, persons, United States Politics and Government, Presidential Election of 2016, Appointments and Executive Changes, Trump, Donald J, 1, 2, 3, 4, N, N, N, N
## 7 subject, persons, persons, subject, organizations, organizations, subject, persons, Presidential Election of 2016, Trump, Donald J, Clinton, Hillary Rodham, Voting and Voters, Democratic Party, Republican Party, United States Politics and Government, Obama, Barack, 2, 4, 5, 6, 7, 8, 9, 10, N, N, N, N, N, N, N, N
## 8 subject, organizations, persons, subject, persons, subject, Presidential Election of 2016, Republican Party, Trump, Donald J, Impeachment, Clinton, Hillary Rodham, Editorials, 1, 2, 3, 4, 5, 6, N, N, N, N, N, N
## 9 subject, persons, persons, Presidential Election of 2016, Chavez, Hugo, Trump, Donald J, 1, 2, 3, N, N, N
## 10 persons, persons, organizations, subject, subject, Wehner, Peter, Trump, Donald J, Republican Party, Presidential Election of 2016, United States Politics and Government, 1, 2, 3, 4, 5, N, N, N, N, N
## pub_date document_type news_desk section_name
## 1 2016-11-09T07:54:21+0000 article Editorial Opinion
## 2 2016-11-09T07:16:23+0000 article OpEd Opinion
## 3 2016-11-10T15:03:59+0000 article OpEd Opinion
## 4 2016-11-08T17:12:34+0000 multimedia U.S.
## 5 2016-11-09T11:05:42+0000 article OpEd Opinion
## 6 2016-11-10T16:21:32+0000 article OpEd Opinion
## 7 2016-11-09T07:28:24+0000 article National U.S.
## 8 2016-11-04T00:26:18+0000 article Editorial Opinion
## 9 2016-11-03T13:01:53+0000 article OpEd Opinion
## 10 2016-11-05T18:30:06+0000 article OpEd Opinion
## byline.original
## 1 <NA>
## 2 By Frank Bruni
## 3 By Clemens Wergin
## 4 By THE NEW YORK TIMES
## 5 By Roger Cohen
## 6 By Eric Posner
## 7 By Matt Flegenheimer and Michael Barbaro
## 8 By The Editorial Board
## 9 By Ioan Grillo
## 10 By Peter Wehner
## byline.person
## 1 NULL
## 2 Frank, NA, Bruni, NA, NA, reported, , 1
## 3 Clemens, NA, Wergin, NA, NA, reported, , 1
## 4 NULL
## 5 Roger, NA, Cohen, NA, NA, reported, , 1
## 6 Eric, NA, Posner, NA, NA, reported, , 1
## 7 Matt, Michael, NA, NA, Flegenheimer, Barbaro, NA, NA, NA, NA, reported, reported, , , 1, 2
## 8 NULL
## 9 Ioan, NA, Grillo, NA, NA, reported, , 1
## 10 Peter, NA, Wehner, NA, NA, reported, , 1
## byline.organization type_of_material
## 1 <NA> Editorial
## 2 <NA> Op-Ed
## 3 <NA> Op-Ed
## 4 The New York Times Video
## 5 <NA> Op-Ed
## 6 <NA> Op-Ed
## 7 <NA> News
## 8 The Editorial Board Editorial
## 9 <NA> Op-Ed
## 10 <NA> Op-Ed
## _id word_count
## 1 nyt://article/5fdad70d-d347-5260-863c-0f7e52d4c700 596
## 2 nyt://article/d711eec5-b9b6-52bd-8c51-8bfda6dfb30e 914
## 3 nyt://article/da090a25-9ba3-5c64-99cd-5c9e2344b92b 921
## 4 nyt://video/bf253029-8ab2-538a-896c-fb4f22c2de03 0
## 5 nyt://article/c6905c70-fefa-5658-8bd3-5c63b0d0c2fe 830
## 6 nyt://article/2b2c29ba-caab-556d-8e9f-ec4fb624c8ba 825
## 7 nyt://article/ea16ce76-d4e5-5902-9584-854ddc7a5948 1613
## 8 nyt://article/0c3a4a9c-afcc-507a-b017-f902e159ae95 568
## 9 nyt://article/d2b18026-f55f-560a-aa8c-a0394759d4f4 936
## 10 nyt://article/f40a0096-413f-52f1-994c-df4123b7b4de 1572
## uri subsection_name
## 1 nyt://article/5fdad70d-d347-5260-863c-0f7e52d4c700 <NA>
## 2 nyt://article/d711eec5-b9b6-52bd-8c51-8bfda6dfb30e <NA>
## 3 nyt://article/da090a25-9ba3-5c64-99cd-5c9e2344b92b <NA>
## 4 nyt://video/bf253029-8ab2-538a-896c-fb4f22c2de03 Politics
## 5 nyt://article/c6905c70-fefa-5658-8bd3-5c63b0d0c2fe <NA>
## 6 nyt://article/2b2c29ba-caab-556d-8e9f-ec4fb624c8ba <NA>
## 7 nyt://article/ea16ce76-d4e5-5902-9584-854ddc7a5948 Politics
## 8 nyt://article/0c3a4a9c-afcc-507a-b017-f902e159ae95 <NA>
## 9 nyt://article/d2b18026-f55f-560a-aa8c-a0394759d4f4 <NA>
## 10 nyt://article/f40a0096-413f-52f1-994c-df4123b7b4de Campaign Stops
##
## $response$meta
## $response$meta$hits
## [1] 388
##
## $response$meta$offset
## [1] 0
##
## $response$meta$time
## [1] 50