Day 3 Scraping the web – extracting data
In Chapter 2 you were shown how to make calls to web pages and get responses. Moreover, you were introduced to making calls to APIs which (usually) give you content in a nice and structured manner. In this chapter, the guiding topic will be how you can extract content from web pages that give you unstructured content in a structured way. The (in our opinion) easiest way to achieve that is by harnessing the way the web is written.
3.1 HTML 101
Web content is usually written in HTML (Hyper Text Markup Language). An HTML document is comprised of elements that are letting its content appear in a certain way.
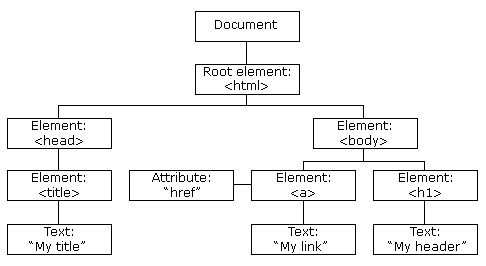
The tree-like structure of an HTML document
The way these elements look is defined by so-called tags.
The opening tag is the name of the element (p
in this case) in angle brackets, and the closing tag is the same with a forward slash before the name. p
stands for a paragraph element and would look like this (since RMarkdown can handle HTML tags, the second line will showcase how it would appear on a web page:
<p> My cat is very grumpy. <p/>
My cat is very grumpy.
The <p>
tag makes sure that the text is standing by itself and that a line break is included thereafter:
<p>My cat is very grumpy</p>. And so is my dog.
would look like this:
My cat is very grumpy
. And so is my dog.
There do exist many types of tags indicating different kinds of elements (about 100). Every page must be in an <html>
element with two children <head>
and <body>
. The former contains the page title and some metadata, the latter the contents you are seeing in your browser. So-called block tags, e.g., <h1>
(heading 1), <p>
(paragraph), or <ol>
(ordered list), structure the page. Inline tags (<b>
– bold, <a>
– link) format text inside block tags.
You can nest elements, e.g., if you want to make certain things bold, you can wrap text in <b>
:
My cat is very grumpy
Then, the <b>
element is considered the child of the <p>
element.
Elements can also bear attributes:
Those attributes will not appear in the actual content. Moreover, they are super-handy for us as scrapers. Here, class
is the attribute name and "editor-note"
the value. Another important attribute is id
. Combined with CSS, they control the appearance of the element on the actual page. A class
can be used by multiple HTML elements whereas an id
is unique.
3.2 Extracting content in rvest
To scrape the web, the first step is to simply read in the web page. rvest
then stores it in the XML format – just another format to store information. For this, we use rvest
’s read_html()
function.
To demonstrate the usage of CSS selectors, I create my own, basic web page using the rvest
function minimal_html()
:
library(rvest)
library(tidyverse)
<- minimal_html('
basic_html <html>
<head>
<title>Page title</title>
</head>
<body>
<h1 id="first">A heading</h1>
<p class="paragraph">Some text & <b>some bold text.</b></p>
<a> Some more <i> italicized text which is not in a paragraph. </i> </a>
<a class="paragraph">even more text & <i>some italicized text.</i></p>
<a id="link" href="www.nyt.com"> The New York Times </a>
</body>
')
basic_html
## {html_document}
## <html>
## [1] <head>\n<meta http-equiv="Content-Type" content="text/html; charset=UTF-8 ...
## [2] <body>\n <h1 id="first">A heading</h1>\n <p class="paragraph">Some ...
#https://htmledit.squarefree.com
CSS is the abbreviation for cascading style sheets and is used to define the visual styling of HTML documents. CSS selectors map elements in the HTML code to the relevant styles in the CSS. Hence, they define patterns that allow us to easily select certain elements on the page. CSS selectors can be used in conjunction with the rvest
function html_elements()
which takes as arguments the read-in page and a CSS selector. Alternatively, you can also provide an XPath which is usually a bit more complicated and will not be covered in this tutorial.
p
selects all<p>
elements.
%>% html_elements(css = "h1") basic_html
## {xml_nodeset (1)}
## [1] <h1 id="first">A heading</h1>
.title
selects all elements that are ofclass
“title”
%>% html_elements(css = ".title") basic_html
## {xml_nodeset (0)}
There are no elements of class
“title”. But some of class
“paragraph”.
%>% html_elements(css = ".paragraph") basic_html
## {xml_nodeset (2)}
## [1] <p class="paragraph">Some text & <b>some bold text.</b></p>
## [2] <a class="paragraph">even more text & <i>some italicized text.</i>\n ...
p.paragraph
analogously takes every<p>
element which is ofclass
“paragraph”.
%>% html_elements(css = "p.paragraph") basic_html
## {xml_nodeset (1)}
## [1] <p class="paragraph">Some text & <b>some bold text.</b></p>
#link
scrapes elements that are ofid
“link”
%>% html_elements(css = "#link") basic_html
## {xml_nodeset (1)}
## [1] <a id="link" href="www.nyt.com"> The New York Times </a>
You can also connect children with their parents by using the combinator. For instance, to extract the italicized text from “a.paragraph,” I can do “a.paragraph i”.
%>% html_elements(css = "a.paragraph i") basic_html
## {xml_nodeset (1)}
## [1] <i>some italicized text.</i>
You can also look at the children by using html_children()
:
%>% html_elements(css = "a.paragraph") %>% html_children() basic_html
## {xml_nodeset (1)}
## [1] <i>some italicized text.</i>
read_html("https://rvest.tidyverse.org") %>%
html_elements("#installation , p")
## {xml_nodeset (9)}
## [1] <p>rvest helps you scrape (or harvest) data from web pages. It is designe ...
## [2] <p>If you’re scraping multiple pages, I highly recommend using rvest in c ...
## [3] <h2 id="installation">Installation<a class="anchor" aria-label="anchor" h ...
## [4] <p>If the page contains tabular data you can convert it directly to a dat ...
## [5] <p>Please note that the rvest project is released with a <a href="https:/ ...
## [6] <p></p>
## [7] <p>Developed by <a href="http://hadley.nz" class="external-link">Hadley W ...
## [8] <p></p>
## [9] <p>Site built with <a href="https://pkgdown.r-lib.org/" class="external-l ...
Unfortunately, web pages in the wild are usually not as easily readable as the small example one I came up with. Hence, I would recommend you to use the SelectorGadget – just drag it into your bookmarks list.
Its usage could hardly be simpler:
- Activate it – i.e., click on the bookmark.
- Click on the content you want to scrape – the things the CSS selector selects will appear green.
- Click on the green things that you don’t want – they will turn red; click on what’s not green yet but what you want – it will turn green.
- copy the CSS selector the gadget provides you with and paste it into the
html_elements()
function.
3.3 Scraping HTML pages with rvest
So far, I have shown you how HTML is written and how to select elements. However, what we want to achieve is extracting the data the elements contained in a proper format and storing it in some sort of tibble. Therefore, we need functions that allow us to grab the data.
The following overview taken from the web scraping cheatsheet shows you the basic “flow” of scraping web pages plus the corresponding functions. In this tutorial, I will limit myself to rvest
functions. Those are of course perfectly compatible with things, for instance, RSelenium
, as long as you feed the content in XML format (i.e., by using read_html()
).
In the first part, I will introduce you to scraping singular pages and extracting their contents. rvest
also allows for proper sessions where you navigate on the web pages and fill out forms. This is to be introduced in the second part.
3.3.1 html_text()
and html_text2()
Extracting text from HTML is easy. You use html_text()
or html_text2()
. The former is faster but will give you not-so-nice results. The latter will give you the text like it would be returned in a web browser.
The following example is taken from the documentation
# To understand the difference between html_text() and html_text2()
# take the following html:
<- minimal_html(
html "<p>This is a paragraph.
This is another sentence.<br>This should start on a new line"
)
# html_text() returns the raw underlying text, which includes white space
# that would be ignored by a browser, and ignores the <br>
%>% html_element("p") %>% html_text() %>% writeLines() html
## This is a paragraph.
## This is another sentence.This should start on a new line
# html_text2() simulates what a browser would display. Non-significant
# white space is collapsed, and <br> is turned into a line break
%>% html_element("p") %>% html_text2() %>% writeLines() html
## This is a paragraph. This is another sentence.
## This should start on a new line
A “real example” would then look like this:
<- read_html("https://en.wikipedia.org/wiki/List_of_current_United_States_senators")
us_senators <- us_senators %>%
text html_elements(css = "p:nth-child(6)") %>%
html_text2()
3.3.2 Extracting tables
The general output format we strive for is a tibble. Oftentimes, data is already stored online in a table format, basically ready for us to analyze them. In the next example, I want to get a table from the Wikipedia page that contains the senators of different States in the United States I have used before. For this first, basic example, I do not use selectors for extracting the right table. You can use rvest::html_table()
. It will give you a list containing all tables on this particular page. We can inspect it using str()
which returns an overview of the list and the tibbles it contains.
<- us_senators %>%
tables html_table()
str(tables)
## List of 22
## $ : tibble [4 × 3] (S3: tbl_df/tbl/data.frame)
## ..$ Affiliation: chr [1:4] "" "" "" "Total"
## ..$ Affiliation: chr [1:4] "Republican Party" "Democratic Party" "Independent" "Total"
## ..$ Members : chr [1:4] "50" "48" "2[a]" "100"
## $ : tibble [11 × 1] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:11] "This article is part of a series on the" "United States Senate" "" "History of the United States Senate" ...
## $ : tibble [2 × 6] (S3: tbl_df/tbl/data.frame)
## ..$ Office : chr [1:2] "President of the Senate[b]" "President pro tempore"
## ..$ Party : chr [1:2] "Democratic" "Democratic"
## ..$ Officer: logi [1:2] NA NA
## ..$ Officer: chr [1:2] "Kamala Harris" "Patrick Leahy"
## ..$ State : chr [1:2] "CA[c]" "VT"
## ..$ Since : chr [1:2] "January 20, 2021" "January 20, 2021Party dean since December 17, 2012"
## $ : tibble [15 × 4] (S3: tbl_df/tbl/data.frame)
## ..$ Office : chr [1:15] "Senate Majority LeaderChair, Senate Democratic Caucus" "Senate Majority Whip" "Senate Assistant Democratic Leader" "Chair, Senate Democratic Policy and Communications Committee" ...
## ..$ Officer: chr [1:15] "Chuck Schumer" "Dick Durbin" "Patty Murray" "Debbie Stabenow" ...
## ..$ State : chr [1:15] "NY" "IL" "WA" "MI" ...
## ..$ Since : chr [1:15] "January 20, 2021Party leader since January 3, 2017" "January 20, 2021Party whip since January 3, 2005" "January 3, 2017" "January 3, 2017" ...
## $ : tibble [9 × 4] (S3: tbl_df/tbl/data.frame)
## ..$ Office : chr [1:9] "Senate Minority Leader" "Senate Minority Whip" "Chair, Senate Republican Conference" "Chair, Senate Republican Policy Committee" ...
## ..$ Officer: chr [1:9] "Mitch McConnell" "John Thune" "John Barrasso" "Roy Blunt" ...
## ..$ State : chr [1:9] "KY" "SD" "WY" "MO" ...
## ..$ Since : chr [1:9] "January 20, 2021Party leader since January 3, 2007" "January 20, 2021Party whip since January 3, 2019" "January 3, 2019" "January 3, 2019" ...
## $ : tibble [100 × 12] (S3: tbl_df/tbl/data.frame)
## ..$ State : chr [1:100] "Alabama" "Alabama" "Alaska" "Alaska" ...
## ..$ Portrait : logi [1:100] NA NA NA NA NA NA ...
## ..$ Senator : chr [1:100] "Richard Shelby" "Tommy Tuberville" "Lisa Murkowski" "Dan Sullivan" ...
## ..$ Party : logi [1:100] NA NA NA NA NA NA ...
## ..$ Party : chr [1:100] "Republican[d]" "Republican" "Republican" "Republican" ...
## ..$ Born : chr [1:100] "(1934-05-06) May 6, 1934 (age 88)" "(1954-09-18) September 18, 1954 (age 67)" "(1957-05-22) May 22, 1957 (age 65)" "(1964-11-13) November 13, 1964 (age 57)" ...
## ..$ Occupation(s) : chr [1:100] "Lawyer" "College football coachPartner, investment management firm" "Lawyer" "U.S. Marine Corps officerLawyerAssistant Secretary of State for Economic and Business Affairs" ...
## ..$ Previous electiveoffice(s): chr [1:100] "U.S. HouseAlabama Senate" "None" "Alaska House of Representatives" "Alaska Attorney General" ...
## ..$ Education : chr [1:100] "University of Alabama (BA, LLB)\nBirmingham School of Law (JD)" "Southern Arkansas University (BS)" "Georgetown University (AB)\nWillamette University (JD)" "Harvard University (AB)\nGeorgetown University (MS, JD)" ...
## ..$ Assumed office : chr [1:100] "January 3, 1987" "January 3, 2021" "December 20, 2002[e]" "January 3, 2015" ...
## ..$ Class : chr [1:100] "2022Class 3" "2026Class 2" "2022Class 3" "2026Class 2" ...
## ..$ Residence[2] : chr [1:100] "Tuscaloosa" "Auburn[3]" "Girdwood" "Anchorage" ...
## $ : tibble [3 × 3] (S3: tbl_df/tbl/data.frame)
## ..$ vteCurrent United States senators: chr [1:3] "President: ▌ Kamala Harris (D) ‧ President pro tempore: ▌ Patrick Leahy (D)" ".mw-parser-output .div-col{margin-top:0.3em;column-width:30em}.mw-parser-output .div-col-small{font-size:90%}.m"| __truncated__ "▌ Republican: 50\n▌ Democratic: 48\n▌ Independent: 2"
## ..$ vteCurrent United States senators: chr [1:3] "President: ▌ Kamala Harris (D) ‧ President pro tempore: ▌ Patrick Leahy (D)" ".mw-parser-output .div-col{margin-top:0.3em;column-width:30em}.mw-parser-output .div-col-small{font-size:90%}.m"| __truncated__ "▌ Republican: 50\n▌ Democratic: 48\n▌ Independent: 2"
## ..$ vteCurrent United States senators: chr [1:3] "President: ▌ Kamala Harris (D) ‧ President pro tempore: ▌ Patrick Leahy (D)" "" "▌ Republican: 50\n▌ Democratic: 48\n▌ Independent: 2"
## $ : tibble [49 × 36] (S3: tbl_df/tbl/data.frame)
## ..$ vteUnited States Congress: chr [1:49] "House of Representatives\nSenate\nJoint session\n(116th ← 117th → 118th)\nLists of United States Congress" "Members and leadersMembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members"| __truncated__ "Members and leaders" "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ ...
## ..$ vteUnited States Congress: chr [1:49] "House of Representatives\nSenate\nJoint session\n(116th ← 117th → 118th)\nLists of United States Congress" "Members and leadersMembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members"| __truncated__ "Members and leaders" "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ ...
## ..$ : chr [1:49] NA "Members and leaders" NA "Membership" ...
## ..$ : chr [1:49] NA "Members and leaders" NA "Members\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseate"| __truncated__ ...
## ..$ : chr [1:49] NA "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ NA "Members" ...
## ..$ : chr [1:49] NA "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ NA "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" ...
## ..$ : chr [1:49] NA "Membership" NA "Senate" ...
## ..$ : chr [1:49] NA "Members\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseate"| __truncated__ NA "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" ...
## ..$ : chr [1:49] NA "Members" NA "House" ...
## ..$ : chr [1:49] NA "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" NA "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ ...
## ..$ : chr [1:49] NA "Senate" NA "Leaders" ...
## ..$ : chr [1:49] NA "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" NA "Senate\nPresident\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Comm"| __truncated__ ...
## ..$ : chr [1:49] NA "House" NA "Senate" ...
## ..$ : chr [1:49] NA "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ NA "President\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Committee Ch"| __truncated__ ...
## ..$ : chr [1:49] NA "Leaders" NA "House" ...
## ..$ : chr [1:49] NA "Senate\nPresident\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Comm"| __truncated__ NA "Speaker\nlist\nLeaders\nBipartisan Legal Advisory Group\nDemocratic Caucus\nRepublican Conference" ...
## ..$ : chr [1:49] NA "Senate" NA "Districts" ...
## ..$ : chr [1:49] NA "President\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Committee Ch"| __truncated__ NA "List\nApportionment\nGerrymandering" ...
## ..$ : chr [1:49] NA "House" NA "Groups" ...
## ..$ : chr [1:49] NA "Speaker\nlist\nLeaders\nBipartisan Legal Advisory Group\nDemocratic Caucus\nRepublican Conference" NA "Congressional caucus\nCaucuses of the United States CongressEthnic and racial\nAfrican-American members\nSenate"| __truncated__ ...
## ..$ : chr [1:49] NA "Districts" NA "Congressional caucus" ...
## ..$ : chr [1:49] NA "List\nApportionment\nGerrymandering" NA "Caucuses of the United States Congress" ...
## ..$ : chr [1:49] NA "Groups" NA "Ethnic and racial" ...
## ..$ : chr [1:49] NA "Congressional caucus\nCaucuses of the United States CongressEthnic and racial\nAfrican-American members\nSenate"| __truncated__ NA "African-American members\nSenate\nHouse\nBlack Caucus\nArab and Middle Eastern members\nAsian Pacific American "| __truncated__ ...
## ..$ : chr [1:49] NA "Congressional caucus" NA "Gender and sexual identity" ...
## ..$ : chr [1:49] NA "Caucuses of the United States Congress" NA "LGBT members\nLGBT Equality Caucus\nWomen\nSenate\nHouse\nIssues Caucus\nCurrent House" ...
## ..$ : chr [1:49] NA "Ethnic and racial" NA "Occupation" ...
## ..$ : chr [1:49] NA "African-American members\nSenate\nHouse\nBlack Caucus\nArab and Middle Eastern members\nAsian Pacific American "| __truncated__ NA "Physicians" ...
## ..$ : chr [1:49] NA "Gender and sexual identity" NA "Religion" ...
## ..$ : chr [1:49] NA "LGBT members\nLGBT Equality Caucus\nWomen\nSenate\nHouse\nIssues Caucus\nCurrent House" NA "Buddhist members\nHindu members\nJewish members\nMormon (LDS) members\nMuslim members" ...
## ..$ : chr [1:49] NA "Occupation" NA "Related" ...
## ..$ : chr [1:49] NA "Physicians" NA "By length of service historically\nCurrent members by wealth\nFrom multiple states\nDied in office\n1790–1899\n"| __truncated__ ...
## ..$ : chr [1:49] NA "Religion" NA NA ...
## ..$ : chr [1:49] NA "Buddhist members\nHindu members\nJewish members\nMormon (LDS) members\nMuslim members" NA NA ...
## ..$ : chr [1:49] NA "Related" NA NA ...
## ..$ : chr [1:49] NA "By length of service historically\nCurrent members by wealth\nFrom multiple states\nDied in office\n1790–1899\n"| __truncated__ NA NA ...
## $ : tibble [16 × 32] (S3: tbl_df/tbl/data.frame)
## ..$ Members and leaders: chr [1:16] "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ "Membership" "Members" "Senate" ...
## ..$ Members and leaders: chr [1:16] "MembershipMembers\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting member"| __truncated__ "Members\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseate"| __truncated__ "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" ...
## ..$ : chr [1:16] "Membership" "Members" NA NA ...
## ..$ : chr [1:16] "Members\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseate"| __truncated__ "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" NA NA ...
## ..$ : chr [1:16] "Members" "Senate" NA NA ...
## ..$ : chr [1:16] "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" NA NA ...
## ..$ : chr [1:16] "Senate" "House" NA NA ...
## ..$ : chr [1:16] "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ NA NA ...
## ..$ : chr [1:16] "House" NA NA NA ...
## ..$ : chr [1:16] "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Leaders" NA NA NA ...
## ..$ : chr [1:16] "Senate\nPresident\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Comm"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Senate" NA NA NA ...
## ..$ : chr [1:16] "President\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Committee Ch"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "House" NA NA NA ...
## ..$ : chr [1:16] "Speaker\nlist\nLeaders\nBipartisan Legal Advisory Group\nDemocratic Caucus\nRepublican Conference" NA NA NA ...
## ..$ : chr [1:16] "Districts" NA NA NA ...
## ..$ : chr [1:16] "List\nApportionment\nGerrymandering" NA NA NA ...
## ..$ : chr [1:16] "Groups" NA NA NA ...
## ..$ : chr [1:16] "Congressional caucus\nCaucuses of the United States CongressEthnic and racial\nAfrican-American members\nSenate"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Congressional caucus" NA NA NA ...
## ..$ : chr [1:16] "Caucuses of the United States Congress" NA NA NA ...
## ..$ : chr [1:16] "Ethnic and racial" NA NA NA ...
## ..$ : chr [1:16] "African-American members\nSenate\nHouse\nBlack Caucus\nArab and Middle Eastern members\nAsian Pacific American "| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Gender and sexual identity" NA NA NA ...
## ..$ : chr [1:16] "LGBT members\nLGBT Equality Caucus\nWomen\nSenate\nHouse\nIssues Caucus\nCurrent House" NA NA NA ...
## ..$ : chr [1:16] "Occupation" NA NA NA ...
## ..$ : chr [1:16] "Physicians" NA NA NA ...
## ..$ : chr [1:16] "Religion" NA NA NA ...
## ..$ : chr [1:16] "Buddhist members\nHindu members\nJewish members\nMormon (LDS) members\nMuslim members" NA NA NA ...
## ..$ : chr [1:16] "Related" NA NA NA ...
## ..$ : chr [1:16] "By length of service historically\nCurrent members by wealth\nFrom multiple states\nDied in office\n1790–1899\n"| __truncated__ NA NA NA ...
## $ : tibble [15 × 12] (S3: tbl_df/tbl/data.frame)
## ..$ X1 : chr [1:15] "Membership" "Members" "Senate" "House" ...
## ..$ X2 : chr [1:15] "Members\nBy length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseate"| __truncated__ "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ ...
## ..$ X3 : chr [1:15] "Members" NA NA NA ...
## ..$ X4 : chr [1:15] "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" NA NA NA ...
## ..$ X5 : chr [1:15] "Senate" NA NA NA ...
## ..$ X6 : chr [1:15] "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" NA NA NA ...
## ..$ X7 : chr [1:15] "House" NA NA NA ...
## ..$ X8 : chr [1:15] "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__ NA NA NA ...
## ..$ X9 : chr [1:15] NA NA NA NA ...
## ..$ X10: chr [1:15] NA NA NA NA ...
## ..$ X11: chr [1:15] NA NA NA NA ...
## ..$ X12: chr [1:15] NA NA NA NA ...
## $ : tibble [3 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:3] "Members" "Senate" "House"
## ..$ X2: chr [1:3] "By length of service\nBy shortness of service\nFreshmen\nYoungest members\nNon-voting members\nUnseated members" "Members\nseniority\nDean\nFormer\nExpelled or censured\nClasses\nBorn outside the U.S.\nResigned\nAppointed\nSwitched parties" "Members\nseniority\nDean\nFormer\nExpelled, censured, and reprimanded\nServed a single term\nSwitched parties\n"| __truncated__
## $ : tibble [2 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:2] "Senate" "House"
## ..$ X2: chr [1:2] "President\nlist\nPresident pro tempore\nlist\nLeaders\nDemocratic Caucus\nChair\nSecretary\nPolicy Committee Ch"| __truncated__ "Speaker\nlist\nLeaders\nBipartisan Legal Advisory Group\nDemocratic Caucus\nRepublican Conference"
## $ : tibble [5 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:5] "Congressional caucus" "Ethnic and racial" "Gender and sexual identity" "Occupation" ...
## ..$ X2: chr [1:5] "Caucuses of the United States Congress" "African-American members\nSenate\nHouse\nBlack Caucus\nArab and Middle Eastern members\nAsian Pacific American "| __truncated__ "LGBT members\nLGBT Equality Caucus\nWomen\nSenate\nHouse\nIssues Caucus\nCurrent House" "Physicians" ...
## $ : tibble [10 × 20] (S3: tbl_df/tbl/data.frame)
## ..$ Powers, privileges, procedure, committees, history, media: chr [1:10] "Powers\nArticle I\nCopyright\nCommerce (Dormant)\nContempt of Congress\nDeclaration of war\nImpeachment\nNatura"| __truncated__ "Powers" "Privileges" "Procedure" ...
## ..$ Powers, privileges, procedure, committees, history, media: chr [1:10] "Powers\nArticle I\nCopyright\nCommerce (Dormant)\nContempt of Congress\nDeclaration of war\nImpeachment\nNatura"| __truncated__ "Article I\nCopyright\nCommerce (Dormant)\nContempt of Congress\nDeclaration of war\nImpeachment\nNaturalization"| __truncated__ "Salaries\nFranking\nImmunity" "Act of Congress\nlist\nAppropriation bill\nBill\nBlue slip\nBudget process\nCensure\nClosed sessions\nHouse\nSe"| __truncated__ ...
## ..$ : chr [1:10] "Powers" NA NA NA ...
## ..$ : chr [1:10] "Article I\nCopyright\nCommerce (Dormant)\nContempt of Congress\nDeclaration of war\nImpeachment\nNaturalization"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "Privileges" NA NA NA ...
## ..$ : chr [1:10] "Salaries\nFranking\nImmunity" NA NA NA ...
## ..$ : chr [1:10] "Procedure" NA NA NA ...
## ..$ : chr [1:10] "Act of Congress\nlist\nAppropriation bill\nBill\nBlue slip\nBudget process\nCensure\nClosed sessions\nHouse\nSe"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "Senate-specific" NA NA NA ...
## ..$ : chr [1:10] "Advice and consent\nClasses\nExecutive communication\nExecutive session\nFilibuster\nJefferson's Manual\nSenate"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "Committees" NA NA NA ...
## ..$ : chr [1:10] "Chairman and ranking member\nOf the Whole\nConference\nDischarge petition\nHearings\nMarkup\nOversight\nList (J"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "Items" NA NA NA ...
## ..$ : chr [1:10] "Gavels\nMace of the House\nSeal of the Senate" NA NA NA ...
## ..$ : chr [1:10] "History" NA NA NA ...
## ..$ : chr [1:10] "House history\nMemoirs\nSpeaker elections\nSenate history\nElection disputes\nMemoirs\nContinental Congress\nFe"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "House history\nMemoirs\nSpeaker elections\nSenate history\nElection disputes\nMemoirs\nContinental Congress\nFe"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "House history\nMemoirs\nSpeaker elections\nSenate history\nElection disputes\nMemoirs\nContinental Congress\nFe"| __truncated__ NA NA NA ...
## ..$ : chr [1:10] "Media" NA NA NA ...
## ..$ : chr [1:10] "C-SPAN\nCongressional Quarterly\nThe Hill\nPolitico\nRoll Call" NA NA NA ...
## $ : tibble [9 × 4] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:9] "Powers" "Privileges" "Procedure" "Senate-specific" ...
## ..$ X2: chr [1:9] "Article I\nCopyright\nCommerce (Dormant)\nContempt of Congress\nDeclaration of war\nImpeachment\nNaturalization"| __truncated__ "Salaries\nFranking\nImmunity" "Act of Congress\nlist\nAppropriation bill\nBill\nBlue slip\nBudget process\nCensure\nClosed sessions\nHouse\nSe"| __truncated__ "Advice and consent\nClasses\nExecutive communication\nExecutive session\nFilibuster\nJefferson's Manual\nSenate"| __truncated__ ...
## ..$ X3: chr [1:9] NA NA NA NA ...
## ..$ X4: chr [1:9] NA NA NA NA ...
## $ : tibble [1 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr "House history\nMemoirs\nSpeaker elections\nSenate history\nElection disputes\nMemoirs\nContinental Congress\nFe"| __truncated__
## ..$ X2: chr "House history\nMemoirs\nSpeaker elections\nSenate history\nElection disputes\nMemoirs\nContinental Congress\nFe"| __truncated__
## $ : tibble [16 × 32] (S3: tbl_df/tbl/data.frame)
## ..$ Capitol Complex (Capitol Hill): chr [1:16] "Legislativeoffices\nCongressional staff\nGov. Accountability Office (GAO)\nComptroller General\nArchitect of th"| __truncated__ "Legislativeoffices" "Offices" "Senate" ...
## ..$ Capitol Complex (Capitol Hill): chr [1:16] "Legislativeoffices\nCongressional staff\nGov. Accountability Office (GAO)\nComptroller General\nArchitect of th"| __truncated__ "Congressional staff\nGov. Accountability Office (GAO)\nComptroller General\nArchitect of the Capitol\nCap. Poli"| __truncated__ "Senate\nCurator\nHistorical\nLibraryHouse\nCongr. Ethics\nEmergency Planning, Preparedness, and Operations\nInt"| __truncated__ "Curator\nHistorical\nLibrary" ...
## ..$ : chr [1:16] "Legislativeoffices" NA "Senate" NA ...
## ..$ : chr [1:16] "Congressional staff\nGov. Accountability Office (GAO)\nComptroller General\nArchitect of the Capitol\nCap. Poli"| __truncated__ NA "Curator\nHistorical\nLibrary" NA ...
## ..$ : chr [1:16] "Offices" NA "House" NA ...
## ..$ : chr [1:16] "Senate\nCurator\nHistorical\nLibraryHouse\nCongr. Ethics\nEmergency Planning, Preparedness, and Operations\nInt"| __truncated__ NA "Congr. Ethics\nEmergency Planning, Preparedness, and Operations\nInterparliamentary Affairs\nLaw Revision Couns"| __truncated__ NA ...
## ..$ : chr [1:16] "Senate" NA NA NA ...
## ..$ : chr [1:16] "Curator\nHistorical\nLibrary" NA NA NA ...
## ..$ : chr [1:16] "House" NA NA NA ...
## ..$ : chr [1:16] "Congr. Ethics\nEmergency Planning, Preparedness, and Operations\nInterparliamentary Affairs\nLaw Revision Couns"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Employees" NA NA NA ...
## ..$ : chr [1:16] "Senate\nSecretary\nChaplain\nCurator\nHistorian\nLibrarian\nPages\nParliamentarian\nSergeant at Arms and Doorke"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Senate" NA NA NA ...
## ..$ : chr [1:16] "Secretary\nChaplain\nCurator\nHistorian\nLibrarian\nPages\nParliamentarian\nSergeant at Arms and Doorkeeper" NA NA NA ...
## ..$ : chr [1:16] "House" NA NA NA ...
## ..$ : chr [1:16] "Chaplain\nChief Administrative Officer\nClerk\nDoorkeeper\nFloor Operations\nFloor Services Chief\nHistorian\nP"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Library ofCongress" NA NA NA ...
## ..$ : chr [1:16] "Congressional Research Service\nReports\nCopyright Office\nRegister of Copyrights\nLaw Library\nPoet Laureate\n"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Gov.Publishing Office" NA NA NA ...
## ..$ : chr [1:16] "Public Printer\nCongressional Pictorial Directory\nCongressional Record\nOfficial Congressional Directory\nU.S."| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Capitol Building" NA NA NA ...
## ..$ : chr [1:16] "Brumidi Corridors\nCongressional Prayer Room\nCrypt\nDome\nStatue of Freedom\nRotunda\nHall of Columns\nStatuar"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Officebuildings" NA NA NA ...
## ..$ : chr [1:16] "Senate\nDirksen\nHart\nMountains and Clouds\nRussellHouse\nBuilding Commission\nCannon\nFord\nLongworth\nO'Neill\nRayburn" NA NA NA ...
## ..$ : chr [1:16] "Senate" NA NA NA ...
## ..$ : chr [1:16] "Dirksen\nHart\nMountains and Clouds\nRussell" NA NA NA ...
## ..$ : chr [1:16] "House" NA NA NA ...
## ..$ : chr [1:16] "Building Commission\nCannon\nFord\nLongworth\nO'Neill\nRayburn" NA NA NA ...
## ..$ : chr [1:16] "Otherfacilities" NA NA NA ...
## ..$ : chr [1:16] "Botanic Garden\nHealth and Fitness Facility\nHouse Recording Studio\nSenate chamber\nOld Senate Chamber\nOld Su"| __truncated__ NA NA NA ...
## ..$ : chr [1:16] "Related" NA NA NA ...
## ..$ : chr [1:16] "Capitol Hill\nUnited States Capitol cornerstone laying" NA NA NA ...
## $ : tibble [15 × 6] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:15] "Legislativeoffices" "Offices" "Senate" "House" ...
## ..$ X2: chr [1:15] "Congressional staff\nGov. Accountability Office (GAO)\nComptroller General\nArchitect of the Capitol\nCap. Poli"| __truncated__ "Senate\nCurator\nHistorical\nLibraryHouse\nCongr. Ethics\nEmergency Planning, Preparedness, and Operations\nInt"| __truncated__ "Curator\nHistorical\nLibrary" "Congr. Ethics\nEmergency Planning, Preparedness, and Operations\nInterparliamentary Affairs\nLaw Revision Couns"| __truncated__ ...
## ..$ X3: chr [1:15] NA "Senate" NA NA ...
## ..$ X4: chr [1:15] NA "Curator\nHistorical\nLibrary" NA NA ...
## ..$ X5: chr [1:15] NA "House" NA NA ...
## ..$ X6: chr [1:15] NA "Congr. Ethics\nEmergency Planning, Preparedness, and Operations\nInterparliamentary Affairs\nLaw Revision Couns"| __truncated__ NA NA ...
## $ : tibble [2 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:2] "Senate" "House"
## ..$ X2: chr [1:2] "Curator\nHistorical\nLibrary" "Congr. Ethics\nEmergency Planning, Preparedness, and Operations\nInterparliamentary Affairs\nLaw Revision Couns"| __truncated__
## $ : tibble [2 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:2] "Senate" "House"
## ..$ X2: chr [1:2] "Secretary\nChaplain\nCurator\nHistorian\nLibrarian\nPages\nParliamentarian\nSergeant at Arms and Doorkeeper" "Chaplain\nChief Administrative Officer\nClerk\nDoorkeeper\nFloor Operations\nFloor Services Chief\nHistorian\nP"| __truncated__
## $ : tibble [2 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ X1: chr [1:2] "Senate" "House"
## ..$ X2: chr [1:2] "Dirksen\nHart\nMountains and Clouds\nRussell" "Building Commission\nCannon\nFord\nLongworth\nO'Neill\nRayburn"
## $ : tibble [2 × 2] (S3: tbl_df/tbl/data.frame)
## ..$ vteOrder of precedence in the United States*: chr [1:2] "PresidentVice President\nGovernor (of the state in which the event is held)\nSpeaker of the House\nChief Justic"| __truncated__ "*not including acting officeholders, visiting dignitaries, auxiliary executive and military personnel and most diplomats"
## ..$ vteOrder of precedence in the United States*: chr [1:2] "PresidentVice President\nGovernor (of the state in which the event is held)\nSpeaker of the House\nChief Justic"| __truncated__ "*not including acting officeholders, visiting dignitaries, auxiliary executive and military personnel and most diplomats"
Here, the table I want is the sixth one. We can grab it by either using double square brackets – [[6]]
– or purrr
’s pluck(6)
.
library(janitor)
<- tables %>%
senators pluck(6)
glimpse(senators)
## Rows: 100
## Columns: 12
## $ State <chr> "Alabama", "Alabama", "Alaska", "Alaska",…
## $ Portrait <lgl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, N…
## $ Senator <chr> "Richard Shelby", "Tommy Tuberville", "Li…
## $ Party <lgl> NA, NA, NA, NA, NA, NA, NA, NA, NA, NA, N…
## $ Party <chr> "Republican[d]", "Republican", "Republica…
## $ Born <chr> "(1934-05-06) May 6, 1934 (age 88)", "(19…
## $ `Occupation(s)` <chr> "Lawyer", "College football coachPartner,…
## $ `Previous electiveoffice(s)` <chr> "U.S. HouseAlabama Senate", "None", "Alas…
## $ Education <chr> "University of Alabama (BA, LLB)\nBirming…
## $ `Assumed office` <chr> "January 3, 1987", "January 3, 2021", "De…
## $ Class <chr> "2022Class 3", "2026Class 2", "2022Class …
## $ `Residence[2]` <chr> "Tuscaloosa", "Auburn[3]", "Girdwood", "A…
## alternative approach using css
<- us_senators %>%
senators html_elements(css = "#senators") %>%
html_table() %>%
pluck(1) %>%
clean_names()
You can see that the tibble contains “dirty” names and that the party column appears twice – which will make it impossible to work with the tibble later on. Hence, I use clean_names()
from the janitor
package to fix that.
3.3.3 Extracting attributes
You can also extract attributes such as links using html_attrs()
. An example would be to extract the headlines and their corresponding links from r-bloggers.com.
<- read_html("https://www.r-bloggers.com") rbloggers
A quick check with the SelectorGadget told me that the element I am looking for is of class “.loop-title” and the child of it is “a”, standing for normal text. With html_attrs()
I can extract the attributes. This gives me a list of named vectors containing the name of the attribute and the value:
<- rbloggers %>% html_elements(css = ".loop-title a")
r_blogger_postings
%>% html_attr("href") r_blogger_postings
## [1] "https://www.r-bloggers.com/2022/06/find-the-maximum-value-by-group-in-r/"
## [2] "https://www.r-bloggers.com/2022/06/servus-es-ist-wieder-soweit-online-shiny-trainings-in-german/"
## [3] "https://www.r-bloggers.com/2022/06/shiny-and-arrow/"
## [4] "https://www.r-bloggers.com/2022/06/how-to-group-and-summarize-data-in-r/"
## [5] "https://www.r-bloggers.com/2022/06/distributions3-user-2022/"
## [6] "https://www.r-bloggers.com/2022/06/what-to-do-and-not-to-do-with-modeling-proportions-fractional-outcomes/"
## [7] "https://www.r-bloggers.com/2022/06/an-introductory-course-in-shiny/"
## [8] "https://www.r-bloggers.com/2022/06/tips-for-rearranging-columns-in-r/"
## [9] "https://www.r-bloggers.com/2022/06/experimenting-with-quarto/"
## [10] "https://www.r-bloggers.com/2022/06/shell-vs-r-fundamentals-from-syntax-to-control-structures-with-zsh-bash/"
## [11] "https://www.r-bloggers.com/2022/06/robservations-33-merging-excel-spreadsheets-with-base-r-and-openxlsx/"
## [12] "https://www.r-bloggers.com/2022/06/how-to-recode-values-in-r/"
## [13] "https://www.r-bloggers.com/2022/06/ropensci-news-digest-june-2022/"
## [14] "https://www.r-bloggers.com/2022/06/user-2022-my-futureverse-profile-parallel-code-slides/"
## [15] "https://www.r-bloggers.com/2022/06/custom-colour-palettes-for-ggplot2/"
## [16] "https://www.r-bloggers.com/2022/06/charting-kaggles-growth-to-10-million-users/"
## [17] "https://www.r-bloggers.com/2022/06/flextable-0-7-2-is-out/"
## [18] "https://www.r-bloggers.com/2022/06/a-shiny-app-to-wrap-blasterjs-and-visualize-ncbi-blast-results-locally/"
## [19] "https://www.r-bloggers.com/2022/06/the-poisson-distribution-from-basic-probability-theory-to-regression-models/"
## [20] "https://www.r-bloggers.com/2022/06/visualizing-the-invasion-of-the-soviet-union-with-luftwaffe-locations/"
Links are stored as attribute “href” – hyperlink reference. html_attr()
allows me to extract the attribute’s value. Hence, building a tibble with the article’s title and its corresponding hyperlink is straight-forward now:
tibble(
title = r_blogger_postings %>% html_text2(),
link = r_blogger_postings %>% html_attr(name = "href")
)
## # A tibble: 20 × 2
## title link
## <chr> <chr>
## 1 Find the Maximum Value by Group in R http…
## 2 Servus, es ist wieder soweit: Online Shiny Trainings in German http…
## 3 Shiny and Arrow http…
## 4 How to Group and Summarize Data in R http…
## 5 distributions3 @ useR! 2022 http…
## 6 What To Do (And Not to Do) with Modeling Proportions/Fractional Outcom… http…
## 7 An introductory course in Shiny http…
## 8 Tips for Rearranging Columns in R http…
## 9 Experimenting with Quarto http…
## 10 Shell vs R Fundamentals – From Syntax to Control Structures with Zsh &… http…
## 11 RObservations #33: Merging Excel Spreadsheets with Base R and openxlsx http…
## 12 How to Recode Values in R http…
## 13 rOpenSci News Digest, June 2022 http…
## 14 useR! 2022: My ‘Futureverse: Profile Parallel Code’ Slides http…
## 15 Custom colour palettes for {ggplot2} http…
## 16 Charting Kaggle’s growth to 10 million users http…
## 17 flextable 0.7.2 is out http…
## 18 A {shiny} app to wrap BlasterJS and visualize NCBI blast results local… http…
## 19 The Poisson distribution: From basic probability theory to regression … http…
## 20 Visualizing the Invasion of the Soviet Union with Luftwaffe Locations http…
Another approach for this would be using the polite
package and its function html_attrs_dfr()
which binds together all the different attributes column-wise and the different elements row-wise.
library(polite)
%>%
rbloggers html_elements(css = ".loop-title a") %>%
html_attrs_dfr() %>%
select(title = 3,
link = 1) %>%
glimpse()
## Rows: 20
## Columns: 2
## $ title <chr> "Find the Maximum Value by Group in R", "Servus, es ist wieder s…
## $ link <chr> "https://www.r-bloggers.com/2022/06/find-the-maximum-value-by-gr…
3.4 Automating scraping
Well, grabbing singular points of data from websites is nice. However, if you want to do things such as collecting large amounts of data or multiple pages, you will not be able to do this without some automation.
An example here would again be the R-bloggers page. It provides you with plenty of R-related content. If you were now eager to scrape all the articles, you would first need to acquire all the different links leading to the blog postings. Hence, you would need to navigate through the site’s pages first to acquire the links.
In general, there are two ways to go about this. The first is to manually create a list of URLs the scraper will visit and take the content you need, therefore not needing to identify where it needs to go next. The other one would be automatically acquiring its next destination from the page (i.e., identifying the “go on” button). Both strategies can also be nicely combined with some sort of session()
.
3.4.1 Looping over pages
For the first approach, we need to check the URLs first. How do they change as we navigate through the pages?
<- "https://www.r-bloggers.com/page/2/"
url_1 <- "https://www.r-bloggers.com/page/3/"
url_2
<- adist(url_1, url_2, counts = TRUE) %>%
initial_dist attr("trafos") %>%
diag() %>%
str_locate_all("[^M]")
str_sub(url_1, start = initial_dist[[1]][1]-5, end = initial_dist[[1]][1]+5) # makes sense for longer urls
## [1] "page/2/"
str_sub(url_2, start = initial_dist[[1]][1]-5, end = initial_dist[[1]][1]+5)
## [1] "page/3/"
There is some sort of underlying pattern and we can harness that. url_1
refers to the second page, url_2
to the third. Hence, if we just combine the basic URL and, say, the numbers from 1 to 10, we could then visit all the pages (exercise 3a) and extract the content we want.
<- str_c("https://www.r-bloggers.com/page/", 1:10, "/") # this is the stringr equivalent of paste()
urls urls
## [1] "https://www.r-bloggers.com/page/1/" "https://www.r-bloggers.com/page/2/"
## [3] "https://www.r-bloggers.com/page/3/" "https://www.r-bloggers.com/page/4/"
## [5] "https://www.r-bloggers.com/page/5/" "https://www.r-bloggers.com/page/6/"
## [7] "https://www.r-bloggers.com/page/7/" "https://www.r-bloggers.com/page/8/"
## [9] "https://www.r-bloggers.com/page/9/" "https://www.r-bloggers.com/page/10/"
You can run this in a for-loop, here’s a quick revision. For the loop to run efficiently, space for every object should be pre-allocated (i.e., you create a list beforehand, and its length can be determined by an educated guess).
## THIS IS PSEUDO CODE!!!
<- vector(mode = "list", length = length(urls)) # pre-allocate space!!!
result_list <-
starting_link for (i in seq_along(urls)){
in urls[[i]]
read in result_list
store page }
3.5 Conclusion
To sum it up: when you have a good research idea that relies on Digital Trace Data that you need to collect, ask yourself the following questions:
- Is there an R package for the web service?
- If 1. == FALSE: Is there an API where I can get the data (if TRUE, use it)
- If 1. == FALSE & 2. == FALSE: Is screen scraping an option and any structure in the data that you can harness?
If you have to rely on screen scraping, also ask yourself the question how you can minimize the number of requests you make to the server. Going back and forth on web pages or navigating through them might not be the best option since it requires multiple requests. The most efficient way is usually to try to get a list of URLs of some sort which you can then just loop over.
3.6 Further links
- More on HTML
- More on CSS selectors
- The
rvest
vignette - A “laymen’s guide” on web scraping (blog post)
3.7 Exercises
- Download all movies from the (IMDb Top250 best movies of all time list)[https://www.imdb.com/chart/top/?ref_=nv_mv_250]. Put them in a tibble with the columns
rank
– in numeric format,title
,url
to IMDb entry,rating
– in numeric format.
Solution. Click to expand!
<- read_html("https://www.imdb.com/chart/top/?ref_=nv_mv_250")
imdb_top250
tibble(
rank = imdb_top250 %>%
html_elements(".titleColumn") %>%
html_text2() %>%
str_extract("^[0-9]+(?=\\.)") %>%
parse_integer(),
title = imdb_top250 %>%
html_elements(".titleColumn a") %>%
html_text2(),
url = imdb_top250 %>%
html_elements(".titleColumn a") %>%
html_attr("href") %>%
str_c("https://www.imdb.com", .),
rating = imdb_top250 %>%
html_elements("strong") %>%
html_text() %>%
parse_double()
)
%>%
imdb_top250 html_elements("a") %>%
html_text2()
- Scrape the (British MPs Wikipedia page)[https://en.wikipedia.org/wiki/List_of_MPs_elected_in_the_2019_United_Kingdom_general_election].
- Scrape the table containing data on all individual MPs. (Hint: you can select and remove single lines using
slice()
, e.g.,tbl %>% slice(1)
retains only the first line,tbl %>% slice(-1)
removes only the first line.) - Extract the links linking to individual pages. Make them readable using
url_absolute()
–url_absolute("/kdfnndfkok", base = "https://wikipedia.com")
–>"https://wikipedia.com/kdfnndfkok"
. Add the links to the table from 1a. - Scrape the textual content of the first MP’s Wikipedia page.
- (ADVANCED!) Wrap 1c. in a function called
scrape_mp_wiki()
.
Solution. Click to expand!
# scrape uk mps
library(tidyverse)
library(rvest)
library(janitor)
#a
<- read_html("https://en.wikipedia.org/wiki/List_of_MPs_elected_in_the_2019_United_Kingdom_general_election") %>%
mp_table html_element("#elected-mps") %>%
html_table() %>%
select(-2, -6) %>%
slice(-651) %>%
clean_names()
#b
<- read_html("https://en.wikipedia.org/wiki/List_of_MPs_elected_in_the_2019_United_Kingdom_general_election") %>%
mp_links html_elements("#elected-mps b a") %>%
html_attr("href")
<- mp_table %>%
table_w_link mutate(link = mp_links %>%
paste0("https://en.wikipedia.org", .))
#c
<- table_w_link %>%
link slice(1) %>%
pull(link)
%>%
link read_html() %>%
html_elements("p") %>%
html_text2() %>%
str_c(collapse = " ") %>%
enframe(name = NULL, value = "wiki_article") %>%
mutate(link = link)
#d
<- function(link){
scrape_page Sys.sleep(0.5)
read_html(link) %>%
html_elements("p") %>%
html_text2() %>%
str_c(collapse = " ") %>%
enframe(name = NULL, value = "wiki_article") %>%
mutate(link = link)
}
<- table_w_link$link[[1]] %>%
mp_wiki_entries map(~{scrape_page(.x)})
<- table_w_link %>%
mp_wiki_entries_tbl left_join(mp_wiki_entries %>% bind_rows())
- Scrape the 10 first pages of R-bloggers in an automated fashion. Make sure to take breaks between requests by including
Sys.sleep(2)
.
- Do so using the url vector we created above.
- Do so by getting the link for the next page and a loop.
- Do so by using
session()
in a loop.
Solution. Click to expand!
# a: check running number in URL, create new URLs, map()
<- str_c("https://www.r-bloggers.com/page/", 1:10, "/")
urls
<- function(url){
scrape_r_bloggers Sys.sleep(2)
read_html(url) %>%
html_elements(css = ".loop-title a") %>%
html_attrs_dfr()
}
map(urls, scrape_r_bloggers)
# b: extract the next page and move forward in the loop
%>% html_elements(css = ".next") %>% html_attr("href")
rbloggers <- "https://www.r-bloggers.com/"
url <- vector(mode = "list", length = 10L)
content
for (i in 1:10){
<- read_html(url)
page
<- page %>%
content[[i]] html_elements(css = ".loop-title a") %>%
html_attrs_dfr()
<- page %>% html_elements(css = ".next") %>% html_attr("href")
url
Sys.sleep(2)
}
# c: session()
session(url) %>% session_follow_link(css = ".next")
<- vector(mode = "list", length = 10L)
content <- session(url)
session_page $response$status_code
session_page
for (i in 1:10){
<- session_page %>%
content[[i]] html_elements(css = ".loop-title a") %>%
html_attrs_dfr()
<- session_page %>% session_follow_link(css = ".next")
session_page }