8.2 Mistake #2: Forgetting Python’s Naming Conventions
= 32 # This won't work!
my variable = 32 # This is preferred!
myVariable = 32 # This works, but is not as common...
MyVariable = 32 # Ditto. my_variable
There is a glaring error in the first line of code above: I inserted a space between the words “my” and “variable”. Unfortunately, this will not fly in Python or in most programming languages - if I do run the code, Python will return a syntax error; I cannot create a variable with the name my variable
(i.e., spaces are not allowed)!
Furthermore, what about this:
= 32
myVariable
print(myvariable)
If I try to run the code as is, Python will return a name error. Here, I am calling the print()
function on myvariable
, yet myvariable
is nowhere to be found in the above code block (hence the reason why Python raised an error). Variables and functions in Python are case sensitive. Since I named my variable myVariable
, I should have typed print(myVariable)
instead of print(myvariable)
!
8.2.1 What can I do to avoid this?
If you are guilty of this mistake, then I think this section of the webpage might do you good. camelCase in particular is probably the way to go for most of your programming in BS1009 (not to worry though, camelCase is easy enough that you can pick it up in a matter of minutes).
Otherwise, if you have a hard time remembering your variables’ names then like I mentioned in the programming tips section, do try and name your variables something intuitive! Furthermore, if you are working in Colab or in an IDE, then there should be an autocomplete function:
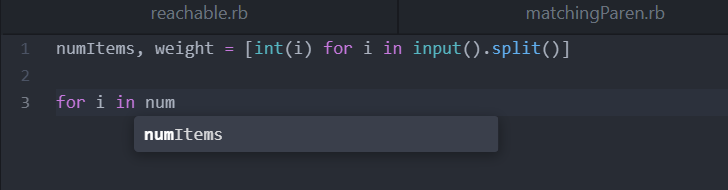
Figure 8.3: Autocomplete Function in Atom
This way, you will not accidentally mistype your variables’ names!